大一c语言模拟自动售货机
时间: 2023-07-09 15:04:42 浏览: 169
好的,下面我为你介绍一下如何用C语言模拟一个自动售货机。
首先,我们需要定义商品的种类和价格。我们可以使用结构体来表示一个商品。例如:
```
struct Product {
char name[20]; // 商品名称
int price; // 商品价格
int stock; // 商品库存
};
```
然后,我们需要定义一个商品数组来存储所有的商品信息。例如:
```
struct Product products[3] = {
{"可乐", 3, 10},
{"雪碧", 3, 10},
{"矿泉水", 2, 10}
};
```
接着,我们需要编写一个函数来显示所有的商品信息。例如:
```
void showProducts() {
printf("编号\t名称\t价格\t库存\n");
for (int i = 0; i < 3; i++) {
printf("%d\t%s\t%d\t%d\n", i+1, products[i].name, products[i].price, products[i].stock);
}
}
```
然后,我们需要编写一个函数来处理用户购买商品的请求。例如:
```
void buyProduct(int index, int amount) {
if (index < 1 || index > 3) {
printf("输入的商品编号不正确!\n");
} else if (amount <= 0) {
printf("输入的购买数量不正确!\n");
} else if (products[index-1].stock < amount) {
printf("该商品库存不足!\n");
} else if (amount * products[index-1].price > balance) {
printf("余额不足!\n");
} else {
products[index-1].stock -= amount;
balance -= amount * products[index-1].price;
printf("购买成功!\n");
}
}
```
最后,我们需要编写一个主函数来实现自动售货机的主要功能。例如:
```
int main() {
int choice;
int amount;
showProducts();
while (1) {
printf("请选择要购买的商品编号(输入0退出):");
scanf("%d", &choice);
if (choice == 0) break;
printf("请输入购买数量:");
scanf("%d", &amount);
buyProduct(choice, amount);
}
return 0;
}
```
以上就是一个简单的自动售货机的C语言模拟代码,希望对你有所帮助。
阅读全文
相关推荐
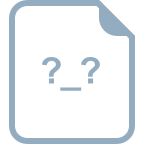
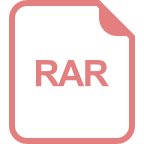
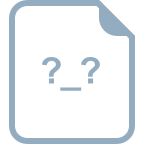
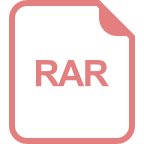
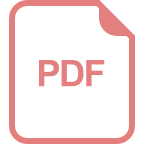
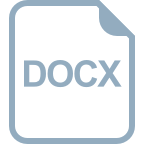
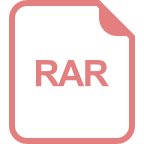
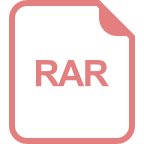
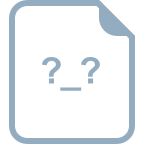
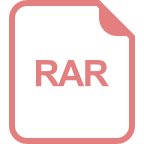
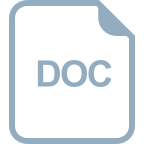