vue写一个到达可视区域触发动画的js
时间: 2023-07-16 12:12:25 浏览: 47
可以使用Intersection Observer实现可视区域触发动画。以下是一个简单的例子:
1. 在HTML中添加需要触发动画的元素,例如:
```
<div class="animate" data-animate="fadeIn">Hello World</div>
```
2. 在CSS中定义动画效果,例如:
```
.animate {
opacity: 0;
transition: opacity 0.5s ease-in-out;
}
.fadeIn {
opacity: 1;
}
```
3. 在Vue组件中添加Intersection Observer,例如:
```
<template>
<div>
<div class="animate" data-animate="fadeIn">Hello World</div>
</div>
</template>
<script>
export default {
mounted() {
const options = {
rootMargin: '0px',
threshold: 0.2
};
const observer = new IntersectionObserver(entries => {
entries.forEach(entry => {
if (entry.isIntersecting) {
const animateClass = entry.target.getAttribute('data-animate');
entry.target.classList.add(animateClass);
observer.unobserve(entry.target);
}
});
}, options);
const elements = document.querySelectorAll('.animate');
elements.forEach(element => {
observer.observe(element);
});
}
};
</script>
```
在这个例子中,Intersection Observer会观察所有class为"animate"的元素。当元素进入可视区域(threshold: 0.2表示元素的20%可见时)时,会添加相应的动画class(例如"fadeIn"),并停止观察该元素,以避免重复触发动画。
注意:Intersection Observer是一个比较新的API,不支持所有浏览器。如果需要兼容旧版浏览器,可以考虑使用Polyfill或其他类似的库。
相关推荐
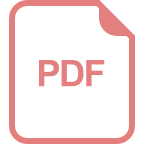
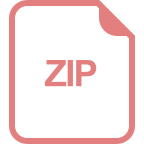
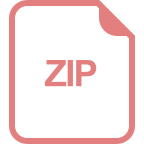














