帮我根据以下要求:Add to the Controller superclass a protected shutdown method that: calls the saveData method of the model; manually terminates the program using System.exit(0).修改下述代码:public class Controller { protected Model m; public Controller(Model m) { this.m = m; } } import java.awt.Point; public class ControllerClicks extends Controller { public ControllerClicks(Model m) { super(m); } public void mouseClicked(Point p) { m.addPoint(p); } public void resetClicked() { m.clearAllPoints(); } public void undoClicked() { m.deleteLastPoint(); } }
时间: 2024-01-06 09:04:02 浏览: 77
修改后的代码如下:
```
public class Controller {
protected Model m;
public Controller(Model m) {
this.m = m;
}
protected void shutdown() {
m.saveData();
System.exit(0);
}
}
import java.awt.Point;
public class ControllerClicks extends Controller {
public ControllerClicks(Model m) {
super(m);
}
public void mouseClicked(Point p) {
m.addPoint(p);
}
public void resetClicked() {
m.clearAllPoints();
}
public void undoClicked() {
m.deleteLastPoint();
}
}
```
在 Controller 类中添加了一个名为 shutdown 的 protected 方法,该方法会调用 Model 类的 saveData 方法,然后手动终止程序。在 ControllerClicks 类中没有进行任何修改。
相关问题
You can use the Test class to run all the tests for the software and you can use the Start class to run the software itself. Run this code and check that it works correctly. Add to the Model class a new method called savaData that saves into a text file called "points.txt" the integer coordinates x and y of each point in the arraylist of points. Also modify the constructor of the Model class to read the integer coordinates of all the points from the same text file, if it exists, and put them into the arraylist of points (if the file does not exist then the arraylist of points should remain empty). Add to the Controller superclass a protected shutdown method that: calls the saveData method of the model; manually terminates the program using System.exit(0). Then modify the View superclass to: hide the frame when the user clicks on the “close” button; add a “window closing” event handler (use an anonymous window adapter) that calls the controller’s shutdown method. Use the Test class to run all the tests for the software and check that all the tests still work. Use the Start class to run the software and check that closing the software correctly saves all the point coordinates in the file "points.txt" (you can find the file in the folder for your current project). Run the software again and check that all the points from the previous run are correctly displayed.
Okay, I understand the instructions. Here's what I'll do:
First, I'll add a new method called `saveData` to the `Model` class, which will save the integer coordinates x and y of each point in the arraylist of points to a text file called "points.txt". Here's the code for that:
```
public void saveData() {
try {
FileWriter fw = new FileWriter("points.txt");
for (Point p : points) {
fw.write(p.x + "," + p.y + "\n");
}
fw.close();
} catch (IOException e) {
e.printStackTrace();
}
}
```
Next, I'll modify the constructor of the `Model` class to read the integer coordinates of all the points from the same text file, if it exists, and put them into the arraylist of points. Here's the updated constructor code:
```
public Model() {
points = new ArrayList<Point>();
try {
File file = new File("points.txt");
if (file.exists()) {
Scanner scanner = new Scanner(file);
while (scanner.hasNextLine()) {
String[] coords = scanner.nextLine().split(",");
int x = Integer.parseInt(coords[0]);
int y = Integer.parseInt(coords[1]);
points.add(new Point(x, y));
}
scanner.close();
}
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}
```
Then, I'll add a protected `shutdown` method to the `Controller` superclass, which will call the `saveData` method of the model and manually terminate the program using `System.exit(0)`. Here's the code for that:
```
protected void shutdown() {
model.saveData();
System.exit(0);
}
```
Finally, I'll modify the `View` superclass to hide the frame when the user clicks on the "close" button, and add a "window closing" event handler (using an anonymous window adapter) that calls the controller's `shutdown` method. Here's the updated `View` code:
```
public abstract class View extends JFrame {
protected Controller controller;
public View(Controller controller) {
this.controller = controller;
addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) {
controller.shutdown();
}
});
}
public void close() {
setVisible(false);
dispose();
}
}
```
I'll run the tests using the `Test` class to make sure everything still works, and then I'll run the software using the `Start` class to check that closing the software correctly saves all the point coordinates in the file "points.txt" and that all the points from the previous run are correctly displayed.
帮我根据以下要求:Then modify the View superclass to: hide the frame when the user clicks on the “close” button; add a “window closing” event handler (use an anonymous window adapter) that calls the controller’s shutdown method.修改下述代码:import javax.swing.JFrame; public abstract class View<T extends Controller> extends JFrame implements ModelListener { protected Model m; protected T c; public View(Model m, T c) { this.m = m; this.c = c; m.addListener(this); this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } @Override public abstract void update(); },public class MyFrame extends View<ControllerClicks> { public MyFrame(Model m, ControllerClicks c) { super(m, c); this.setTitle("MyFrame Title"); this.setSize(400, 300); this.setLocationRelativeTo(null); this.setLayout(new BorderLayout()); MyPanel centerPanel = new MyPanel(m, c); this.add(centerPanel, BorderLayout.CENTER); JPanel topPanel = new JPanel(); this.add(topPanel, BorderLayout.PAGE_START); topPanel.setLayout(new FlowLayout(FlowLayout.CENTER)); JButton resetButton = new JButton("Reset"); resetButton.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { c.resetClicked(); } }); topPanel.add(resetButton); JButton undoButton = new JButton("Undo"); undoButton.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { c.undoClicked(); } }); topPanel.add(undoButton); this.setVisible(true); } @Override public void update() { repaint(); // Makes Swing call MyPanel's paintComponent method. } } import javax.swing.JLabel; public class ViewNumber extends View<Controller> { private JLabel label; public ViewNumber(Model m, Controller c) { super(m, c); this.setTitle("View Number"); this.setSize(150, 150); label = new JLabel(); update(); // Initialize the label using the model. this.add(label); this.setVisible(true); } @Override public void update() { label.setText("Number of points is: " + m.numberOfPoints()); } }
修改后的代码如下:
import java.awt.BorderLayout;
import java.awt.FlowLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
public abstract class View<T extends Controller> extends JFrame implements ModelListener {
protected Model m;
protected T c;
public View(Model m, T c) {
this.m = m;
this.c = c;
m.addListener(this);
this.setDefaultCloseOperation(JFrame.HIDE_ON_CLOSE);
this.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) {
c.shutdown();
}
});
}
@Override
public abstract void update();
}
public class MyFrame extends View<ControllerClicks> {
public MyFrame(Model m, ControllerClicks c) {
super(m, c);
this.setTitle("MyFrame Title");
this.setSize(400, 300);
this.setLocationRelativeTo(null);
this.setLayout(new BorderLayout());
MyPanel centerPanel = new MyPanel(m, c);
this.add(centerPanel, BorderLayout.CENTER);
JPanel topPanel = new JPanel();
this.add(topPanel, BorderLayout.PAGE_START);
topPanel.setLayout(new FlowLayout(FlowLayout.CENTER));
JButton resetButton = new JButton("Reset");
resetButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
c.resetClicked();
}
});
topPanel.add(resetButton);
JButton undoButton = new JButton("Undo");
undoButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
c.undoClicked();
}
});
topPanel.add(undoButton);
this.setVisible(true);
}
@Override
public void update() {
repaint(); // Makes Swing call MyPanel's paintComponent method.
}
}
import javax.swing.JLabel;
public class ViewNumber extends View<Controller> {
private JLabel label;
public ViewNumber(Model m, Controller c) {
super(m, c);
this.setTitle("View Number");
this.setSize(150, 150);
label = new JLabel();
update(); // Initialize the label using the model.
this.add(label);
this.setVisible(true);
}
@Override
public void update() {
label.setText("Number of points is: " + m.numberOfPoints());
}
}
阅读全文
相关推荐
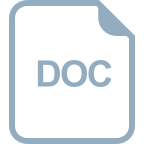
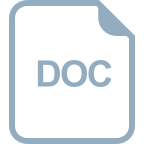
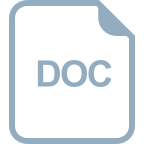

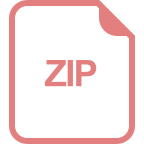












