根据以下要求写出java代码:The isIn method indicates whether the point at coordinates (x, y) (which are the arguments of the method) is currently inside the bubble or not. The edge of the bubble counts as being inside of the bubble. HINT: use Pythagoras's theorem to compute the distance from the center of the bubble to the point (x, y). The draw method uses the graphics object g to draw the bubble. HINT: remember that the color of the graphics object g is changed in the draw method of the superclass of Bubble. Also add a testBubble method to test all your methods (including inherited methods, but excluding the isVisible method, which I provide, and excluding the draw method since it requires as argument a graphics object g that you do not currently have)
时间: 2023-07-15 08:11:23 浏览: 44
```java
import java.awt.Color;
import java.awt.Graphics;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class Bubble extends JPanel {
private int x, y, radius;
public Bubble(int x, int y, int radius, Color color) {
super();
setLocation(x, y);
setRadius(radius);
setColor(color);
}
public boolean isIn(int x, int y) {
int dx = this.x - x;
int dy = this.y - y;
int distance = (int) Math.sqrt(dx * dx + dy * dy);
return distance <= radius;
}
public void draw(Graphics g) {
g.setColor(getColor());
g.fillOval(x - radius, y - radius, 2 * radius, 2 * radius);
}
public static void testBubble() {
Bubble b1 = new Bubble(100, 100, 50, Color.RED);
Bubble b2 = new Bubble(200, 200, 75, Color.BLUE);
System.out.println("b1.isIn(120, 120) = " + b1.isIn(120, 120));
System.out.println("b2.isIn(200, 250) = " + b2.isIn(200, 250));
}
// Getters and setters
public int getX() {
return x;
}
public void setX(int x) {
this.x = x;
}
public int getY() {
return y;
}
public void setY(int y) {
this.y = y;
}
public int getRadius() {
return radius;
}
public void setRadius(int radius) {
this.radius = radius;
}
private Color color;
public Color getColor() {
return color;
}
public void setColor(Color color) {
this.color = color;
}
}
```
相关推荐
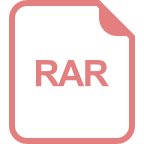
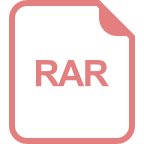
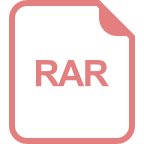
















