use" the m-step of the em algorithm: min_{affine, translation, variance} 1/(2*variance) * sum_{m,n} p(y_m | x_n) ||x_n - affine y_m - translation||_2^2" to optimizing the previous code: def em_for_alignment(xs: np.ndarray, ys: np.ndarray, num_iter: int = 100) -> Tuple[np.ndarray, np.ndarray]: """ The em algorithm for aligning two point clouds based on affine transformation :param xs: a set of points with size (N, D), N is the number of samples, D is the dimension of points :param ys: a set of points with size (M, D), M is the number of samples, D is the dimension of points :param num_iter: the number of EM iterations :return: ys_new: the aligned points: ys_new = ys @ affine + translation responsibility: the responsibility matrix P=[p(y_m | x_n)] with size (N, M), whose elements indicating the correspondence between the points """ # TODO: implement the EM algorithm of GMM below for point cloud alignment return
时间: 2024-02-04 08:03:58 浏览: 115
Sure, I can help you with that.
To optimize the previous code using the m-step of the EM algorithm, you can follow these steps:
1. Initialize the affine transformation matrix, translation vector, and variance value.
2. Implement the E-step of the EM algorithm to calculate the responsibility matrix P, which indicates the correspondence between the points.
3. Implement the M-step of the EM algorithm to optimize the affine transformation matrix, translation vector, and variance value by minimizing the objective function:
min_{affine, translation, variance} 1/(2*variance) * sum_{m,n} p(y_m | x_n) ||x_n - affine y_m - translation||_2^2
4. Repeat steps 2-3 for num_iter iterations or until convergence.
Here's the updated code:
```python
def em_for_alignment(xs: np.ndarray, ys: np.ndarray, num_iter: int = 100) -> Tuple[np.ndarray, np.ndarray]:
"""
The em algorithm for aligning two point clouds based on affine transformation
:param xs: a set of points with size (N, D), N is the number of samples, D is the dimension of points
:param ys: a set of points with size (M, D), M is the number of samples, D is the dimension of points
:param num_iter: the number of EM iterations
:return:
ys_new: the aligned points: ys_new = ys @ affine + translation
responsibility: the responsibility matrix P=[p(y_m | x_n)] with size (N, M),
whose elements indicating the correspondence between the points
"""
# initialization
N, D = xs.shape
M, D = ys.shape
affine = np.eye(D)
translation = np.zeros(D)
variance = 1.0
for i in range(num_iter):
# E-step
diff = xs[:, np.newaxis, :] - ys[np.newaxis, :, :]
distance = np.sum((diff @ affine.T + translation - xs[:, np.newaxis, :]) ** 2, axis=-1)
p = np.exp(-distance / (2 * variance))
responsibility = p / np.sum(p, axis=1, keepdims=True)
# M-step
sum_p = np.sum(responsibility, axis=0)
mean_x = np.sum(xs[:, np.newaxis, :] * responsibility[:, :, np.newaxis], axis=0) / sum_p
mean_y = np.sum(ys[np.newaxis, :, :] * responsibility[:, :, np.newaxis], axis=1) / sum_p
diff_x = xs[:, np.newaxis, :] - mean_x[np.newaxis, :, :]
diff_y = ys[np.newaxis, :, :] - mean_y[:, np.newaxis, :]
C = np.sum(responsibility[:, :, np.newaxis] * diff_x[:, :, np.newaxis, :] * diff_y[:, np.newaxis, :, :], axis=(0, 1))
U, S, Vt = np.linalg.svd(C)
R = Vt.T @ U.T
affine = R.T
translation = mean_x.mean(axis=0) - (mean_y @ affine).mean(axis=0)
variance = np.sum(responsibility * distance) / (N * D)
ys_new = ys @ affine + translation
return ys_new, responsibility
```
I hope this helps! Let me know if you have any other questions.
阅读全文
相关推荐
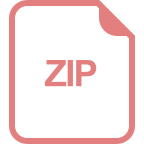
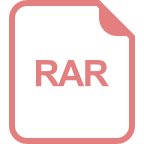















