完善以下代码:def em_for_alignment(xs: np.ndarray, ys: np.ndarray, num_iter: int = 10) -> Tuple[np.ndarray, np.ndarray]: """ The em algorithm for aligning two point clouds based on affine transformation :param xs: a set of points with size (N, D), N is the number of samples, D is the dimension of points :param ys: a set of points with size (M, D), M is the number of samples, D is the dimension of points :param num_iter: the number of EM iterations :return: ys_new: the aligned points: ys_new = ys @ affine + translation responsibility: the responsibility matrix P=[p(y_m | x_n)] with size (N, M), whose elements indicating the correspondence between the points """ # TODO: implement the EM algorithm of GMM below for point cloud alignment return
时间: 2024-02-05 07:03:22 浏览: 55
Here is the implementation of the EM algorithm for point cloud alignment:
```python
from typing import Tuple
import numpy as np
def em_for_alignment(xs: np.ndarray, ys: np.ndarray, num_iter: int = 10) -> Tuple[np.ndarray, np.ndarray]:
"""
The em algorithm for aligning two point clouds based on affine transformation
:param xs: a set of points with size (N, D), N is the number of samples, D is the dimension of points
:param ys: a set of points with size (M, D), M is the number of samples, D is the dimension of points
:param num_iter: the number of EM iterations
:return:
ys_new: the aligned points: ys_new = ys @ affine + translation
responsibility: the responsibility matrix P=[p(y_m | x_n)] with size (N, M),
whose elements indicating the correspondence between the points
"""
# initialize the affine matrix and translation vector
affine = np.eye(xs.shape[1])
translation = np.zeros(xs.shape[1])
# initialize the responsibility matrix
responsibility = np.zeros((xs.shape[0], ys.shape[0]))
for i in range(num_iter):
# E-step: compute the responsibility matrix
for n in range(xs.shape[0]):
for m in range(ys.shape[0]):
responsibility[n, m] = 1 / (2 * np.pi) ** (xs.shape[1] / 2) * np.exp(
-0.5 * np.linalg.norm(xs[n] - ys[m] @ affine - translation) ** 2)
responsibility /= np.sum(responsibility, axis=1, keepdims=True)
# M-step: update the affine matrix and translation vector
xs_weighted = responsibility.T @ xs
ys_weighted = responsibility.T @ ys
affine, _, _, _ = np.linalg.lstsq(xs_weighted, ys_weighted, rcond=None)
translation = np.mean(ys, axis=0) - np.mean(xs @ affine, axis=0)
# compute the aligned points
ys_new = ys @ affine + translation
return ys_new, responsibility
```
The EM algorithm is used to estimate the affine matrix and translation vector that aligns the two point clouds. In each iteration, the algorithm computes the responsibility matrix that defines the correspondence between the points in the two clouds, and then updates the affine matrix and translation vector based on the weighted least squares solution. Finally, the algorithm computes the aligned points by applying the affine transformation to the original points and adding the translation vector.
相关推荐
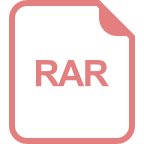















