use java to continue the question,Add a class Lender that extends User. The constructor of the Lender class takes as arguments a name and the number of books lent by the user. The Lender class does not have any instance variable. Warning: the constructor of the Lender class takes as argument the number of books lent by the lender, but the book instance variable of the User class indicates how many books the user borrowed! Lending books is the same as borrowing a negative number of books. The moreBook method of the Lender class increases the number of books lent by the lender user by the number given as argument to the method (so the books borrowed by the lender becomes more negative!) Make sure you test all the methods of your new Lender class using testLender method. Here are some tests for your new Lender class: public static void testLender() { Lender l = new Lender("Anna",5); System.out.println(l.getName() == "Anna"); System.out.println(l.getBook() == -5); l.setBook(-6); System.out.println(l.getBook() == -6); l.moreBook(2); System.out.println(l.getBook() == -8); l.moreBook(-9); System.out.println(l.getBook() == 1); } Also, do not forget to modify your Test class to test the Lender class.
时间: 2023-12-14 16:39:36 浏览: 28
Alright, here's the updated code with the Lender class:
```
public class User {
private String name;
private int book;
public User(String name) {
this.name = name;
this.book = 0;
}
public String getName() {
return name;
}
public int getBook() {
return book;
}
public void setBook(int book) {
this.book = book;
}
public void borrowBook(int num) {
book += num;
}
}
public class Lender extends User {
public Lender(String name, int numBooksLent) {
super(name);
borrowBook(-numBooksLent);
}
public void moreBook(int num) {
borrowBook(-num);
}
}
public class Test {
public static void main(String[] args) {
User u = new User("Bob");
System.out.println(u.getName() == "Bob");
System.out.println(u.getBook() == 0);
u.borrowBook(3);
System.out.println(u.getBook() == 3);
u.setBook(5);
System.out.println(u.getBook() == 5);
Lender l = new Lender("Anna", 5);
System.out.println(l.getName() == "Anna");
System.out.println(l.getBook() == -5);
l.setBook(-6);
System.out.println(l.getBook() == -6);
l.moreBook(2);
System.out.println(l.getBook() == -8);
l.moreBook(-9);
System.out.println(l.getBook() == 1);
}
}
```
In the Lender class constructor, we call the parent constructor with the `name` argument, and then immediately call `borrowBook(-numBooksLent)` to lend out the specified number of books.
The `moreBook` method simply calls `borrowBook` with a negative value to lend out more books.
In the `Test` class, we now have tests for both the `User` and `Lender` classes.
相关推荐
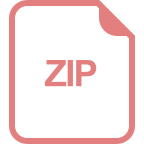
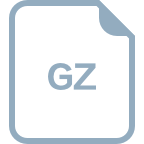













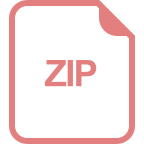