Note that, even if a consumer is a borrower, the readPosInt method prevents the typed number of books from being negative. This means a borrower will never throw a NotALenderException. Nevertheless the code of the main method of your CLI class must handle this exception by printing the error message "BUG! This must never happen!" and immediately terminating the program using System.exit(1).Javacase实现
时间: 2023-06-27 10:04:33 浏览: 56
以下是一个可能的Javacase实现,用于处理上述情况:
```java
public class CLI {
public static void main(String[] args) {
try {
Scanner scanner = new Scanner(System.in);
System.out.println("Welcome to the library!");
System.out.print("Are you a lender or a borrower? ");
String input = scanner.nextLine().trim().toLowerCase();
if (input.equals("lender")) {
System.out.print("Enter the number of books you want to lend: ");
int numBooks = readPosInt(scanner);
Lender lender = new Lender(numBooks);
System.out.println("You have successfully registered as a lender.");
// do something with lender object
} else if (input.equals("borrower")) {
System.out.print("Enter the number of books you want to borrow: ");
int numBooks = readPosInt(scanner);
Borrower borrower = new Borrower(numBooks);
System.out.println("You have successfully registered as a borrower.");
// do something with borrower object
} else {
System.out.println("Invalid input. Please try again.");
}
} catch (NotALenderException e) {
System.out.println("BUG! This must never happen!");
System.exit(1);
}
}
public static int readPosInt(Scanner scanner) throws NotALenderException {
int numBooks = scanner.nextInt();
if (numBooks < 0) {
throw new NotALenderException("Number of books cannot be negative.");
}
return numBooks;
}
}
```
该实现包括一个名为`readPosInt`的辅助方法,该方法使用`Scanner`对象读取正整数,并在输入为负数时引发`NotALenderException`。在`main`方法中,我们捕获该异常并立即终止程序。
相关推荐
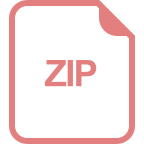
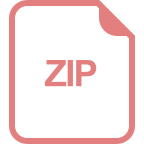
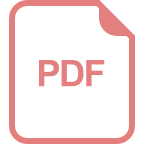














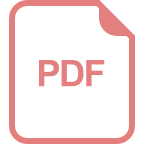