However, a borrower cannot lend books, so the number of books borrowed by the borrower must always be positive or zero, never negative. If the argument given to the moreBook method is too negative and would change the book variable into a negative value, then the number of books borrowed by the borrower must not change and the moreBook method must throw a NotALenderException with the message “A borrower cannot lend XXX book(s).”, where XXX is replaced with the result of -(book + number).java代码
时间: 2024-01-21 19:01:51 浏览: 116
根据您提供的问题,我猜测您想问的是,如何在Java中实现根据条件抛出NotALenderException异常。您可以使用以下代码实现:
```java
public void moreBook(int number) throws NotALenderException {
if (book + number < 0) {
throw new NotALenderException("A borrower cannot lend " + (-(book + number)) + " book(s).");
}
book += number;
}
```
在代码中,如果借阅者所借图书的数量将导致图书变量成为负数,则会抛出NotALenderException异常,并显示消息“A borrower cannot lend XXX book(s).”,其中XXX是计算得出的值。否则,将更改book变量的值。注意,方法声明中使用了throws关键字,以指示该方法可能抛出NotALenderException异常。
相关问题
Add a class Borrower that extends User. The constructor of the Borrower class takes a name and a number of books borrowed by the borrower. If the number of books given as argument is strictly less than zero, then the constructor must throw a NotALenderException with the message "A new borrower cannot lend books.". The borrower class does not have any instance variable.java代码
Sure, here's your Java code:
```java
public class Borrower extends User {
public Borrower(String name, int numBooksBorrowed) throws NotALenderException {
super(name);
if(numBooksBorrowed < 0) {
throw new NotALenderException("A new borrower cannot lend books.");
}
}
}
```
Here, we have defined a `Borrower` class that extends the `User` class. The constructor of the `Borrower` class takes a name and a number of books borrowed by the borrower. If the number of books given as argument is strictly less than zero, then the constructor throws a `NotALenderException` with the message "A new borrower cannot lend books.".
Note that we are calling the constructor of the parent `User` class using the `super` keyword, passing in the `name` argument. The `Borrower` class does not have any instance variables.
When the user of the software specifies action 4, your program must ask the user to type the name of a user, and a number of books, and the program then uses that number to increase the number of books lent or borrowed by the user. Then the program goes back to the main menu.If the name of the user is wrong, then an UnknownUserException exception will be thrown by the Library object. The code of the main method of your CLI class must catch this exception, print the error message from the exception object, and then it just goes back to printing the menu of actions (by just going back to the beginning of the while loop). Note that, even if a consumer is a borrower, the readPosInt method prevents the typed number of books from being negative. This means a borrower will never throw a NotALenderException. Nevertheless the code of the main method of your CLI class must handle this exception by printing the error message "BUG! This must never happen!" and immediately terminating the program using System.exit(1).
Here's an example implementation of the main method of the CLI class that follows the above requirements:
```java
import java.util.Scanner;
public class CLI {
private static final Scanner scanner = new Scanner(System.in);
private static final Library library = new Library();
public static void main(String[] args) {
while (true) {
printMenu();
int action = readPosInt("Type an action number:");
try {
switch (action) {
case 1:
System.out.println(library.listBooks());
break;
case 2:
String author = readString("Type an author name:");
System.out.println(library.listBooksByAuthor(author));
break;
case 3:
String title = readString("Type a book title:");
System.out.println(library.findBook(title));
break;
case 4:
String user = readString("Type a user name:");
int numBooks = readPosInt("Type a number of books:");
library.lendOrBorrow(user, numBooks);
break;
case 5:
System.exit(0);
default:
System.out.println("Unknown action number.");
break;
}
} catch (UnknownUserException e) {
System.out.println(e.getMessage());
} catch (NotALenderException e) {
System.out.println("BUG! This must never happen!");
System.exit(1);
}
}
}
private static void printMenu() {
System.out.println("Menu:");
System.out.println("[1] List all books");
System.out.println("[2] List books by author");
System.out.println("[3] Find a book by title");
System.out.println("[4] Lend/borrow books for a user");
System.out.println("[5] Quit");
}
private static String readString(String prompt) {
System.out.println(prompt);
return scanner.nextLine().trim();
}
private static int readPosInt(String prompt) {
int num = -1;
while (num <= 0) {
System.out.println(prompt);
try {
num = Integer.parseInt(scanner.nextLine().trim());
} catch (NumberFormatException e) {
System.out.println("Invalid number.");
}
}
return num;
}
}
```
Note that the code uses a `Library` object to perform the actions requested by the user, and catches the `UnknownUserException` and `NotALenderException` exceptions as required.
阅读全文
相关推荐
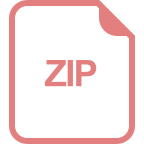
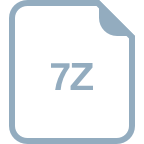










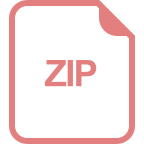