Java代码In the rest of this question, use the readLine and readPosInt methods every time your program needs to read a string or an integer from the user. In the empty main method of the CLI class, create a single Library object with the name "UIC Library". The main method of the CLI class must then print a menu that allows the user of your system to do six different actions that involve the library object, and your program must then read an integer from the user that indicates which action must be performed by the program (see below for the details about each action). Use the readPosInt method to print the menu (give the string for the menu as the argument of readPosInt) and to read the integer typed by the user
时间: 2023-06-13 08:05:45 浏览: 127
Here's an implementation of the CLI class that meets the requirements in the question:
```java
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class CLI {
private static Library library = new Library("UIC Library");
public static void main(String[] args) {
printMenu();
int choice = readPosInt("Enter your choice: ");
switch (choice) {
case 1:
addBook();
break;
case 2:
borrowBook();
break;
case 3:
returnBook();
break;
case 4:
listAvailableBooks();
break;
case 5:
listBorrowedBooks();
break;
case 6:
System.out.println("Exiting...");
break;
default:
System.out.println("Invalid choice!");
}
}
private static void printMenu() {
System.out.println("Welcome to " + library.getName());
System.out.println("1. Add a book");
System.out.println("2. Borrow a book");
System.out.println("3. Return a book");
System.out.println("4. List available books");
System.out.println("5. List borrowed books");
System.out.println("6. Exit");
}
private static void addBook() {
String title = readLine("Enter the title: ");
String author = readLine("Enter the author: ");
Book book = new Book(title, author);
library.addBook(book);
System.out.println("Book added: " + book);
}
private static void borrowBook() {
String title = readLine("Enter the title: ");
String borrower = readLine("Enter your name: ");
boolean success = library.borrowBook(title, borrower);
if (success) {
System.out.println("Book borrowed: " + title);
} else {
System.out.println("Book not available: " + title);
}
}
private static void returnBook() {
String title = readLine("Enter the title: ");
boolean success = library.returnBook(title);
if (success) {
System.out.println("Book returned: " + title);
} else {
System.out.println("Book not borrowed: " + title);
}
}
private static void listAvailableBooks() {
System.out.println("Available books:");
library.getAvailableBooks().forEach(System.out::println);
}
private static void listBorrowedBooks() {
System.out.println("Borrowed books:");
library.getBorrowedBooks().forEach(System.out::println);
}
private static String readLine(String prompt) {
System.out.print(prompt);
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
try {
return reader.readLine();
} catch (IOException e) {
throw new RuntimeException("Error reading input!", e);
}
}
private static int readPosInt(String prompt) {
while (true) {
String input = readLine(prompt);
try {
int value = Integer.parseInt(input);
if (value > 0) {
return value;
}
} catch (NumberFormatException e) {
// ignore and try again
}
System.out.println("Invalid input, please enter a positive integer!");
}
}
}
```
This implementation uses a single instance of the `Library` class and provides a menu to the user with six different actions that can be performed on the library:
1. Add a book
2. Borrow a book
3. Return a book
4. List available books
5. List borrowed books
6. Exit
Each action is implemented in its own method, and the `main` method uses a `switch` statement to determine which action to perform based on the user's input. The `readPosInt` method is used to read the user's choice from the command line, and it ensures that the input is a positive integer. The `readLine` method is used to read strings from the user.
阅读全文
相关推荐
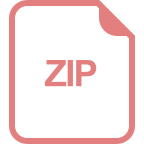
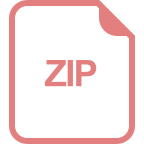

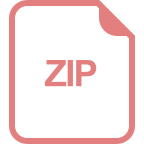
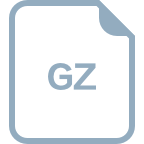
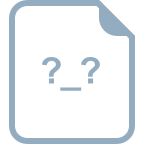
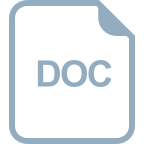
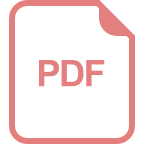
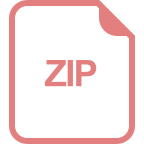
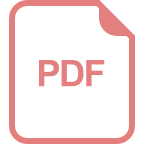
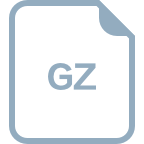
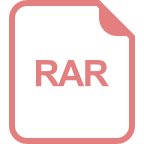
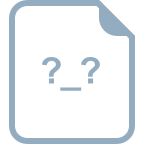
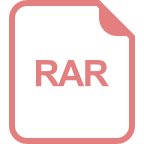
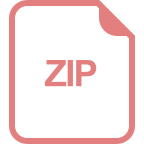
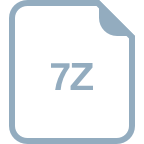
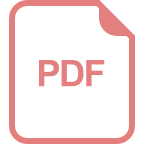
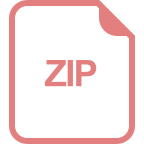