write java/The moreBook method takes the name of a user and a number of books (as a string) as arguments. The moreBook method of the controller then transforms the number of books from a string to an integer (using the Integer.parseInt static method) and calls the moreBook method of the library to increase the number of books borrowed or lent by the user (depending on what kind of user it is) of a specific user, by the given argument.
时间: 2023-06-29 16:04:55 浏览: 85
Here is a sample implementation of the moreBook method in Java:
```
public class LibraryController {
private Library library;
public LibraryController(Library library) {
this.library = library;
}
public void moreBook(String userName, String numBooks) {
int books = Integer.parseInt(numBooks);
User user = library.getUser(userName);
if (user instanceof Borrower) {
library.moreBorrowedBooks((Borrower) user, books);
} else if (user instanceof Lender) {
library.moreLentBooks((Lender) user, books);
}
}
}
public class Library {
// other methods
public void moreBorrowedBooks(Borrower borrower, int numBooks) {
borrower.setNumBorrowedBooks(borrower.getNumBorrowedBooks() + numBooks);
}
public void moreLentBooks(Lender lender, int numBooks) {
lender.setNumLentBooks(lender.getNumLentBooks() + numBooks);
}
}
public abstract class User {
private String name;
public User(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
public class Borrower extends User {
private int numBorrowedBooks;
public Borrower(String name, int numBorrowedBooks) {
super(name);
this.numBorrowedBooks = numBorrowedBooks;
}
public int getNumBorrowedBooks() {
return numBorrowedBooks;
}
public void setNumBorrowedBooks(int numBorrowedBooks) {
this.numBorrowedBooks = numBorrowedBooks;
}
}
public class Lender extends User {
private int numLentBooks;
public Lender(String name, int numLentBooks) {
super(name);
this.numLentBooks = numLentBooks;
}
public int getNumLentBooks() {
return numLentBooks;
}
public void setNumLentBooks(int numLentBooks) {
this.numLentBooks = numLentBooks;
}
}
```
In this implementation, the LibraryController class has a moreBook method that takes a user name and a number of books as arguments. The method first parses the number of books from a string to an integer using the Integer.parseInt static method. It then gets the user object from the library based on the provided user name.
Depending on what kind of user the user object is (a Borrower or a Lender), the method calls the moreBorrowedBooks or moreLentBooks method of the library to increase the number of books borrowed or lent by the user by the given argument.
The Library class has methods for increasing the number of borrowed or lent books by a user. The User class is an abstract class that represents a library user, and the Borrower and Lender classes are concrete implementations of User that represent users who borrow or lend books, respectively.
相关推荐















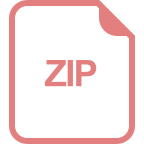