When the user then clicks on the button, the action listener of the button must read the name of the library user that was typed in the first text field (using the getText method of the text field) and the number of books that was typed in the second text field (using again the getText method) and must call the moreBook method of the controller with these two strings as arguments. The moreBook method of the controller then returns a string as result. If the string returned by the moreBook method of the controller is different from the empty string "" then this string must be displayed back to the user using a message dialog (using the showMessageDialog method of the JOptionPane class). If the string returned by the moreBook method of the controller is equal to the empty string "" then nothing happens in ViewMoreBook. The update method of the ViewMoreBook class does nothing, because the ViewMoreBook class does not graphically display any data from the library (the model).java代码
时间: 2023-06-26 16:07:24 浏览: 55
以下是实现上述功能的 Java 代码:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class ViewMoreBook extends JFrame {
private JTextField nameField;
private JTextField numField;
private JButton moreButton;
private Controller controller;
public ViewMoreBook(Controller controller) {
this.controller = controller;
// Set up the UI components
nameField = new JTextField(20);
numField = new JTextField(10);
moreButton = new JButton("More Books");
// Add action listener to the button
moreButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String name = nameField.getText();
String num = numField.getText();
String result = controller.moreBook(name, num);
if (!result.equals("")) {
JOptionPane.showMessageDialog(null, result);
}
}
});
// Set up the layout of the UI
JPanel panel = new JPanel(new FlowLayout());
panel.add(new JLabel("Name:"));
panel.add(nameField);
panel.add(new JLabel("Number of Books:"));
panel.add(numField);
panel.add(moreButton);
this.setContentPane(panel);
this.pack();
this.setTitle("View More Books");
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
public void update() {
// Do nothing in this method
}
}
```
在这个代码中,我们创建了一个名为 ViewMoreBook 的类,它继承自 JFrame 类,用于实现图形用户界面(GUI)。我们在构造函数中初始化了 UI 组件,并且为 moreButton 按钮添加了一个 action listener。在 action listener 中,我们调用了 getText 方法从文本框中读取名字和书籍数量。然后,我们调用了 controller 的 moreBook 方法,并将返回值存储为 result。如果 result 不为空字符串,则使用 JOptionPane 的 showMessageDialog 方法将其显示给用户。
最后,我们定义了 update 方法,该方法并不真正更新 ViewMoreBook,因为它不需要显示来自模型的数据。
相关推荐
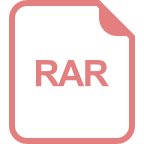
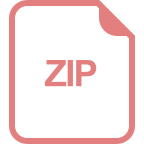
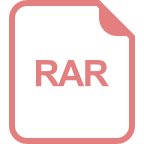














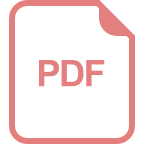
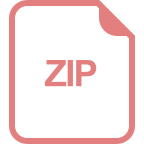