continue to use Java language, Add a class Borrower that extends User. The constructor of the Borrower class takes a name and a number of books borrowed by the borrower. If the number of books given as argument is strictly less than zero, then the constructor must throw a NotALenderException with the message “A new borrower cannot lend books.”. The borrower class does not have any instance variable. The moreBook method of the Borrower class increases the number of books borrowed by the borrower by the number of books given as argument to the method (so the books borrowed by the borrower becomes more positive!) For example, if a borrower currently borrows 10 books and moreBook(2) is called then the borrower borrows 12 books. It is fine for the moreBook method to be given a negative value as argument, which means the borrower then just returned some books. For example, if a borrower currently borrows 10 books and moreBook(-2) is called then the borrower borrows 8 books. However, a borrower cannot lend books, so the number of books borrowed by the borrower must always be positive or zero, never negative. If the argument given to the moreBook method is too negative and would change the book variable into a negative value, then the number of books borrowed by the borrower must not change and the moreBook method must throw a NotALenderException with the message “A borrower cannot lend XXX book(s).”, where XXX is replaced with the result of -(book + number). For example, if a borrower currently borrows 10 books and moreBook(-12) is called then the borrower still borrows 10 books and the method throws a NotALenderException with the message “A borrower cannot lend 2 book(s).”. Note: to simplify the project, do not worry about the setBook method. Change other classes and interfaces as necessary
时间: 2023-12-14 20:39:46 浏览: 82
Here is the updated code with the Borrower class:
```java
public class NotALenderException extends Exception {
public NotALenderException(String message) {
super(message);
}
}
public class User {
private String name;
public User(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
public class Borrower extends User {
private int booksBorrowed;
public Borrower(String name, int booksBorrowed) throws NotALenderException {
super(name);
if (booksBorrowed < 0) {
throw new NotALenderException("A new borrower cannot lend books.");
}
this.booksBorrowed = booksBorrowed;
}
public void moreBook(int number) throws NotALenderException {
int newBooksBorrowed = booksBorrowed + number;
if (newBooksBorrowed < 0) {
throw new NotALenderException("A borrower cannot lend " + (-newBooksBorrowed) + " book(s).");
}
booksBorrowed = newBooksBorrowed;
}
public int getBooksBorrowed() {
return booksBorrowed;
}
}
```
In this updated code, the Borrower class extends the User class and has an additional instance variable `booksBorrowed` which represents the number of books borrowed by the borrower. The constructor of the Borrower class takes a name and a number of books borrowed by the borrower. If the number of books given as argument is strictly less than zero, then a NotALenderException is thrown. The moreBook method of the Borrower class increases or decreases the number of books borrowed by the borrower by the number of books given as argument to the method. If the argument given to the moreBook method is too negative and would change the book variable into a negative value, then a NotALenderException is thrown. The getBooksBorrowed method returns the number of books borrowed by the borrower.
阅读全文
相关推荐
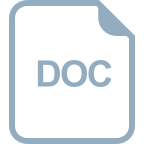
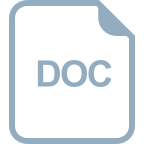
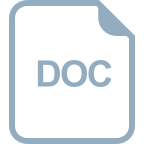













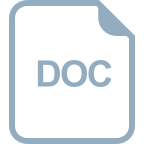
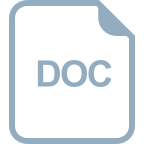
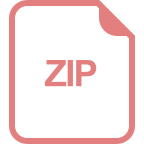
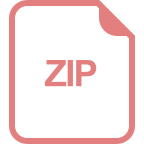