write java。The create method takes as arguments the name of a new library user, a number of books (as a string), and an integer representing the role of user to create (where the integer 0 means a lender and the integer 1 means a borrower). The create method of the controller then transforms the book number from a string to an integer (using the Integer.parseInt static method), creates an object from the correct class (based on the role specified by the user input: lender or borrower) and calls the addUser method of the library to add the new user object to the library. • If no exception occurs then the create method of the controller returns the empty string. • If the constructor of the Borrower class throws a NotALenderException then the create method of the controller must catch this exception and return as result the error message from the exception object. • If the parseInt method of the Integer class throws a NumberFormatException (because the user typed something which is not an integer) then the create method of the controller must catch this exception and return as result the error message from the exception object.
时间: 2023-12-03 11:47:31 浏览: 77
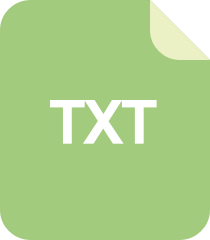
java对象创建过程

Here is a possible implementation of the `create` method in Java:
```java
public class LibraryController {
private Library library;
public LibraryController(Library library) {
this.library = library;
}
public String create(String name, String bookString, int role) {
try {
int bookCount = Integer.parseInt(bookString);
User user;
if (role == 0) {
user = new Lender(name, bookCount);
} else if (role == 1) {
user = new Borrower(name, bookCount);
} else {
return "Invalid role: " + role;
}
library.addUser(user);
return "";
} catch (NotALenderException e) {
return e.getMessage();
} catch (NumberFormatException e) {
return "Invalid book count: " + bookString;
}
}
}
```
In this implementation, the `create` method takes three arguments: the name of the new user, the book count as a string, and the role as an integer. The method first tries to parse the book count using `Integer.parseInt`, and if successful, creates a user object based on the role specified by the user input. If the role is not 0 or 1, an error message is returned.
If the user object is successfully created, the method calls the `addUser` method of the library to add the new user object to the library. If no exception occurs, an empty string is returned.
If the constructor of the `Borrower` class throws a `NotALenderException`, the method catches the exception and returns the error message from the exception object.
If the `parseInt` method of the `Integer` class throws a `NumberFormatException` (because the user typed something which is not an integer), the method catches the exception and returns an error message indicating that the book count is invalid.
阅读全文
相关推荐
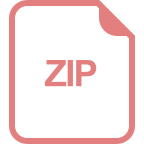
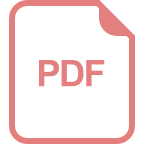




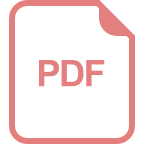
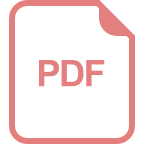
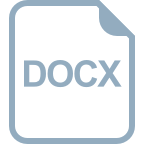
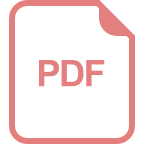
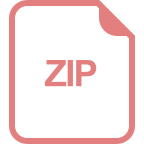
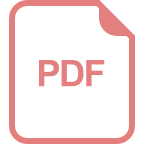
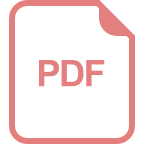
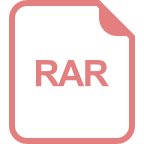
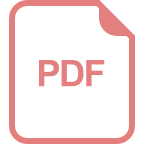
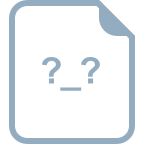
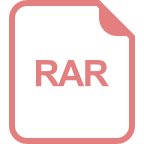