write java。The moreBook method takes the name of a user and a number of books (as a string) as arguments. The moreBook method of the controller then transforms the number of books from a string to an integer (using the Integer.parseInt static method) and calls the moreBook method of the library to increase the number of books borrowed or lent by the user (depending on what kind of user it is) of a specific user, by the given argument. • If no exception occurs then the moreBook method of the controller returns the empty string. • If the moreBook method of the library throws an UnknownUserException then the moreBook method of the controller must catch this exception and return as result the error message from the exception object. • If the moreBook method of the library throws a NotALenderException then the moreBook method of the controller must catch this exception and return as result the error message from the exception object. • If the parseInt method of the Integer class throws a NumberFormatException (because the user typed something which is not an integer) then the moreBook method of the controller must catch this exception and return as result the error message from the exception object.
时间: 2023-12-03 16:46:50 浏览: 36
Here is the Java code for the described functionality:
```
public class LibraryController {
private Library library;
public LibraryController(Library library) {
this.library = library;
}
public String moreBook(String userName, String numBooks) {
try {
int num = Integer.parseInt(numBooks);
library.moreBook(userName, num);
return "";
} catch (UnknownUserException e) {
return e.getMessage();
} catch (NotALenderException e) {
return e.getMessage();
} catch (NumberFormatException e) {
return e.getMessage();
}
}
}
public class Library {
private Map<String, User> users;
public Library() {
users = new HashMap<>();
}
public void moreBook(String userName, int numBooks) throws UnknownUserException, NotALenderException {
User user = users.get(userName);
if (user == null) {
throw new UnknownUserException("Unknown user: " + userName);
}
if (!(user instanceof Lender)) {
throw new NotALenderException(userName + " is not a lender");
}
((Lender) user).moreBooks(numBooks);
}
}
public class User {
private String name;
public User(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
public class Lender extends User {
private int numBooks;
public Lender(String name) {
super(name);
numBooks = 0;
}
public int getNumBooks() {
return numBooks;
}
public void moreBooks(int num) {
numBooks += num;
}
}
public class UnknownUserException extends Exception {
public UnknownUserException(String message) {
super(message);
}
}
public class NotALenderException extends Exception {
public NotALenderException(String message) {
super(message);
}
}
```
The LibraryController class has a constructor that takes a Library object as a parameter. The moreBook method takes a user name and a number of books as arguments. It uses the Integer.parseInt static method to parse the number of books from a string to an integer. It then calls the moreBook method of the Library object to increase the number of books borrowed or lent by the user, depending on what kind of user it is.
If no exception occurs, the moreBook method of the controller returns the empty string. If the moreBook method of the library throws an UnknownUserException, the moreBook method of the controller catches this exception and returns the error message from the exception object. If the moreBook method of the library throws a NotALenderException, the moreBook method of the controller catches this exception and returns the error message from the exception object. If the parseInt method of the Integer class throws a NumberFormatException, the moreBook method of the controller catches this exception and returns the error message from the exception object.
The Library class has a map of users, where the key is the user name and the value is a User object. The moreBook method takes a user name and a number of books as arguments. It throws an UnknownUserException if the user is not found in the map, and a NotALenderException if the user is not an instance of the Lender class. Otherwise, it calls the moreBooks method of the Lender object to increase the number of books borrowed by the user.
The User class has a name field and a getName method.
The Lender class extends the User class and adds a numBooks field and a moreBooks method.
The UnknownUserException and NotALenderException classes extend the Exception class and have constructors that take an error message as a parameter.
相关推荐















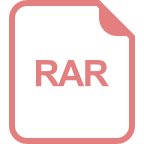
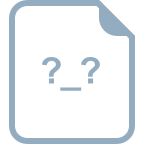
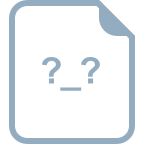
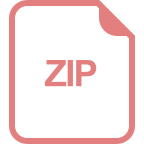