Action 2: adding a new user to the library. When the user of the software specifies action 2, your program must add a new user to the library. To add a new user, your program needs to ask the user three things: the role of user (an integer read using readPosInt: the integer 1 represents lender, the integer 2 represents borrower, any other integer must result in an error message "Unknown user role!" being printed and the software going immediately back to the main menu), the name of the user (a string read using readLine), and the initial number of books that the user lends (for a lender) or borrows (for a borrower). You program must then create the correct user, add it to the library, and print an information message. The program then goes back to the menu.
时间: 2023-11-22 14:55:08 浏览: 73
Here is the code for adding a new user to the library:
```
def add_user(library):
role = readPosInt("Enter the role of the user (1 for lender, 2 for borrower): ")
if role == 1:
user_role = "Lender"
elif role == 2:
user_role = "Borrower"
else:
print("Unknown user role!")
return
name = readLine("Enter the name of the user: ")
num_books = readPosInt(f"Enter the initial number of books {name} lends (for a lender) or borrows (for a borrower): ")
if user_role == "Lender":
user = Lender(name, num_books)
else:
user = Borrower(name, num_books)
library.add_user(user)
print(f"User {name} ({user_role}) added to the library.")
```
Here, we first ask the user for the role of the new user, their name, and the initial number of books they lend or borrow. If the role is not 1 or 2, we print an error message and return to the main menu.
Otherwise, we create a new user object based on their role and add it to the library. We print a message confirming the addition of the new user to the library and return to the main menu.
阅读全文
相关推荐
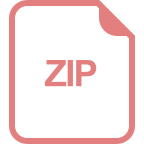
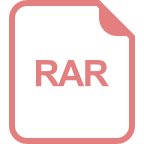


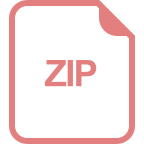









