verilog HDL输出三角波 正弦波 方波 脉冲波
时间: 2023-08-05 10:02:04 浏览: 364
下面是 Verilog HDL 实现输出三角波、正弦波、方波和脉冲波的例子:
三角波:
```
module triangle_wave(
input clk,
input rst,
output reg out
);
reg [7:0] count;
initial begin
count <= 0;
out <= 0;
end
always @(posedge clk or posedge rst) begin
if (rst) begin
count <= 0;
out <= 0;
end else begin
if (count == 8'hFF) begin
count <= 0;
end else begin
count <= count + 1;
end
if (count <= 8'h7F) begin
out <= count;
end else begin
out <= ~count + 1;
end
end
end
endmodule
```
正弦波:
```
module sine_wave(
input clk,
input rst,
output reg signed [7:0] out
);
reg [7:0] count;
initial begin
count <= 0;
out <= 0;
end
always @(posedge clk or posedge rst) begin
if (rst) begin
count <= 0;
out <= 0;
end else begin
count <= count + 1;
out <= $signed(64.0 + 64.0 * $sin(count * 2.0 * $pi / 256.0));
end
end
endmodule
```
方波:
```
module square_wave(
input clk,
input rst,
output reg out
);
reg [7:0] count;
initial begin
count <= 0;
out <= 0;
end
always @(posedge clk or posedge rst) begin
if (rst) begin
count <= 0;
out <= 0;
end else begin
if (count == 8'hFF) begin
count <= 0;
out <= ~out;
end else begin
count <= count + 1;
end
end
end
endmodule
```
脉冲波:
```
module pulse_wave(
input clk,
input rst,
output reg out
);
reg [7:0] count;
initial begin
count <= 0;
out <= 0;
end
always @(posedge clk or posedge rst) begin
if (rst) begin
count <= 0;
out <= 0;
end else begin
if (count == 8'h7F) begin
count <= 0;
out <= 1;
end else begin
count <= count + 1;
out <= 0;
end
end
end
endmodule
```
这些例子中,计数器的位宽和计数的范围可以根据需要进行调整。同时,三角波和正弦波的输出信号使用了有符号的变量,需要使用 `$signed` 函数进行转换。
阅读全文
相关推荐
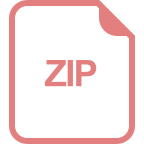
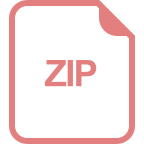
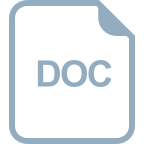
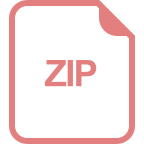
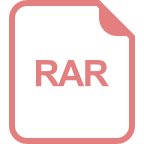
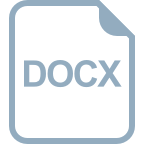
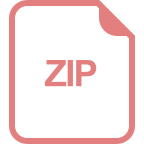
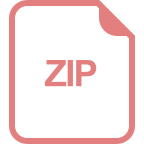
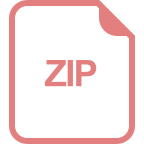