写一个java接口。接受一个map类型的参数,将map根据key-value 存入文件夹中 ,多次请求也都存在同一个 文件夹中
时间: 2024-04-09 20:29:15 浏览: 91
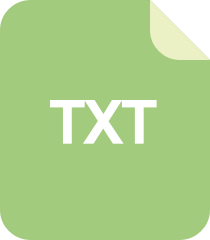
解密后的字符串截取放map中
您可以通过以下方式编写一个Java接口,接收一个Map类型的参数,并将Map根据key-value存入同一个文件夹中:
```java
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.util.Map;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class MapToFileController {
private static final String FOLDER_PATH = "your_folder_path";
@PostMapping("/saveMapToFile")
public String saveMapToFile(@RequestBody Map<String, String> map) {
try {
File folder = new File(FOLDER_PATH);
folder.mkdirs(); // 创建文件夹
// 生成一个唯一的文件名
String fileName = generateFileName();
// 创建文件,并将Map写入文件
File file = new File(folder, fileName);
FileWriter writer = new FileWriter(file);
for (Map.Entry<String, String> entry : map.entrySet()) {
writer.write(entry.getKey() + "=" + entry.getValue() + "\n");
}
writer.close();
return "Map saved to file: " + file.getAbsolutePath();
} catch (IOException e) {
e.printStackTrace();
return "Error saving map to file.";
}
}
private String generateFileName() {
// 这里可以根据需要生成一个唯一的文件名,比如使用时间戳或随机数等
return "map_file.txt";
}
}
```
上述代码使用Spring框架编写了一个RestController,其中的`saveMapToFile`方法接收一个Map类型的参数,并将Map中的key-value对写入指定文件夹中的文件中。
在`FOLDER_PATH`常量中,请替换为您想要存储文件的文件夹路径。
当您向`/saveMapToFile`接口发送POST请求,并在请求体中传递一个Map参数时,该Map将被写入文件夹中的一个文件中。每次请求都将存储在同一个文件夹中,但会生成不同的文件名,以保证数据不被覆盖。
请注意,此示例仅提供了基本的文件写入功能,您可以根据实际需求进行修改和扩展。
阅读全文
相关推荐
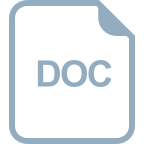
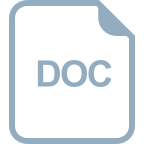















