某商品出售按购买数量打折,如果购买数量超过60个则按6折销售,51到60之间按7折销售,41到50之间按8折销售,31到40之间按9折销售。30及以下按原价出售,输入商品数量和商品单价(元),输出所购商品的总价。用C语言写一个程序
时间: 2023-08-31 14:32:27 浏览: 31
### 回答1:
#include <stdio.h>
int main() {
int num; // 商品数量
float price; // 商品单价
float total_price; // 总价
printf("请输入商品数量和商品单价(以空格隔开):\n");
scanf("%d %f", &num, &price);
if (num > 60) {
total_price = num * price * 0.6;
} else if (num >= 51 && num <= 60) {
total_price = num * price * 0.7;
} else if (num >= 41 && num <= 50) {
total_price = num * price * 0.8;
} else if (num >= 31 && num <= 40) {
total_price = num * price * 0.9;
} else {
total_price = num * price;
}
printf("所购商品的总价为:%.2f 元\n", total_price);
return 0;
}
### 回答2:
#include<stdio.h>
int main() {
int quantity;
float price;
float total;
printf("请输入商品数量:");
scanf("%d", &quantity);
printf("请输入商品单价(元):");
scanf("%f", &price);
if (quantity > 60) {
total = quantity * price * 0.6;
} else if(quantity > 50){
total = quantity * price * 0.7;
} else if(quantity > 40){
total = quantity * price * 0.8;
} else if(quantity > 30){
total = quantity * price * 0.9;
} else {
total = quantity * price;
}
printf("所购商品的总价为:%.2f元\n", total);
return 0;
}
### 回答3:
以下是一个用C语言编写的程序,用于计算购买某商品的总价:
```c
#include <stdio.h>
int main() {
int quantity;
float unitPrice;
float totalPrice;
printf("请输入商品数量:");
scanf("%d", &quantity);
printf("请输入商品单价(元):");
scanf("%f", &unitPrice);
if (quantity > 60) {
totalPrice = quantity * unitPrice * 0.6;
} else if (quantity >= 51) {
totalPrice = quantity * unitPrice * 0.7;
} else if (quantity >= 41) {
totalPrice = quantity * unitPrice * 0.8;
} else if (quantity >= 31) {
totalPrice = quantity * unitPrice * 0.9;
} else {
totalPrice = quantity * unitPrice;
}
printf("所购商品的总价为:%.2f元\n", totalPrice);
return 0;
}
```
运行程序后,会要求输入商品数量和商品单价,并自动计算出所购商品的总价。根据题目中的条件,程序根据购买数量进行了不同的折扣计算。最后,程序将打印出所购商品的总价。
注意:程序中使用了浮点数(`float`类型)来存储单价和总价,以保留小数点后两位。如果需要更精确的计算结果,可以使用`double`类型代替`float`类型。
相关推荐
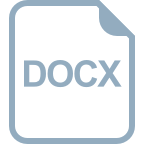
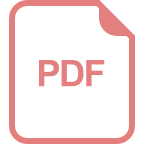
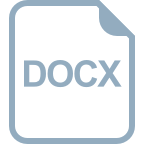















