studio弹窗代码
时间: 2023-07-23 07:17:24 浏览: 94
如果你需要在代码中弹出退出确认窗口,可以使用以下代码:
```
import javax.swing.JOptionPane;
int result = JOptionPane.showConfirmDialog(null, "确定要退出吗?", "确认", JOptionPane.YES_NO_OPTION);
if (result == JOptionPane.YES_OPTION) {
System.exit(0);
}
```
这段代码会弹出一个确认窗口,询问用户是否要退出程序。如果用户点击了 "确定" 按钮,则程序会调用 System.exit(0) 方法退出。如果用户点击了 "取消" 按钮,则程序会继续执行。
相关问题
android studio 弹窗
Android Studio是一款由Google开发的集成开发环境(IDE),用于开发Android应用程序。在Android Studio中,弹窗是一种常见的用户界面元素,用于显示一些提示信息、警告或者需要用户进行选择的对话框。
在Android Studio中,可以通过以下方式创建和使用弹窗:
1. AlertDialog:AlertDialog是Android提供的一种常用的弹窗类型,可以用于显示简单的提示信息、警告或者需要用户进行选择的对话框。可以通过AlertDialog.Builder类来创建AlertDialog,并设置标题、消息内容、按钮等属性。
示例代码:
```
AlertDialog.Builder builder = new AlertDialog.Builder(context);
builder.setTitle("提示");
builder.setMessage("这是一个弹窗示例");
builder.setPositiveButton("确定", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
// 点击确定按钮后的操作
}
});
builder.setNegativeButton("取消", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
// 点击取消按钮后的操作
}
});
AlertDialog dialog = builder.create();
dialog.show();
```
2. Toast:Toast是一种轻量级的弹窗,用于显示短暂的提示信息。可以通过Toast类来创建Toast弹窗,并设置显示的文本内容和显示时长。
示例代码:
```
Toast.makeText(context, "这是一个Toast示例", Toast.LENGTH_SHORT).show();
```
以上是Android Studio中创建和使用弹窗的两种常见方式。除了AlertDialog和Toast,还可以使用其他第三方库或自定义View来实现更复杂的弹窗效果。
Android studio实现listview弹窗代码
可以通过以下代码实现:
1.在xml文件中定义ListView
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:padding="10dp">
<TextView
android:layout_width="0dp"
android:layout_weight="1"
android:layout_height="wrap_content"
android:text="Select Option" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Select"
android:id="@+id/btn_select_option"/>
</LinearLayout>
2.在activity文件中使用AlertDialog弹出ListView窗口
public class MainActivity extends AppCompatActivity {
String[] myList = {"Option 1", "Option 2", "Option 3", "Option 4"};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button btnSelectOption = (Button) findViewById(R.id.btn_select_option);
btnSelectOption.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
AlertDialog.Builder builder = new AlertDialog.Builder(MainActivity.this);
builder.setTitle("Select option");
builder.setItems(myList, new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Toast.makeText(MainActivity.this, myList[which], Toast.LENGTH_LONG).show();
}
});
AlertDialog dialog = builder.create();
dialog.show();
}
});
}
}
在弹出的对话框中,用户可以选择其中一个选项,然后通过Toast显示选择的选项。
阅读全文
相关推荐
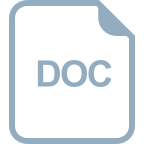
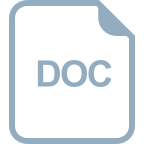
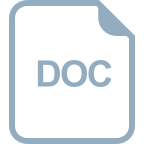





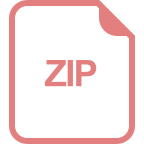







