java判断字符串相等
时间: 2023-10-31 07:26:36 浏览: 22
可以使用equals()方法来判断字符串相等,例如:
String str1 = "Hello";
String str2 = "World";
if(str1.equals(str2)){
System.out.println("字符串相等");
}else{
System.out.println("字符串不相等");
}
输出结果为"字符串不相等"。
相关问题
java 判断字符串相等
在 Java 中,判断字符串相等需要使用 equals 方法,例如:
```java
String s1 = "hello";
String s2 = "world";
if (s1.equals(s2)) {
System.out.println("s1 equals s2");
} else {
System.out.println("s1 not equals s2");
}
```
需要注意的是,如果使用 == 操作符判断字符串相等,实际上比较的是两个字符串对象的引用是否相同,而不是字符串的内容是否相同。
java字符串判断相等_java判断字符串是否相等的方法
Java中判断字符串是否相等有以下几种方法:
1. 使用equals()方法判断字符串是否相等
```java
String s1 = "hello";
String s2 = "world";
if (s1.equals(s2)) {
System.out.println("s1 is equal to s2");
} else {
System.out.println("s1 is not equal to s2");
}
```
2. 使用equalsIgnoreCase()方法判断字符串是否相等,不区分大小写
```java
String s1 = "Hello";
String s2 = "hello";
if (s1.equalsIgnoreCase(s2)) {
System.out.println("s1 is equal to s2");
} else {
System.out.println("s1 is not equal to s2");
}
```
3. 使用compareTo()方法判断字符串是否相等,返回值为0表示相等
```java
String s1 = "hello";
String s2 = "hello";
if (s1.compareTo(s2) == 0) {
System.out.println("s1 is equal to s2");
} else {
System.out.println("s1 is not equal to s2");
}
```
注意:以上方法中,使用“==”判断两个字符串是否相等是不正确的,应该使用equals()方法来判断。
阅读全文
相关推荐
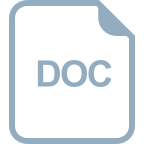
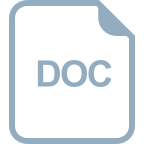
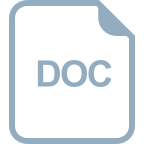
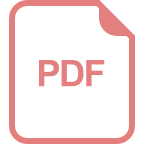










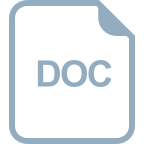
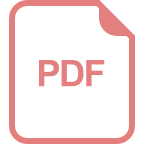
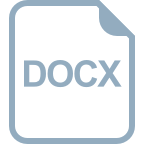