设计点的类,点有坐标(x,y)属性,并为这个点类设置一个方法来计算两点间的距离。给出完整代码
时间: 2023-09-04 21:04:21 浏览: 181
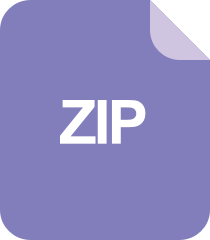
基于Java求三维立体空间中两点的距离【100011982】
### 回答1:
以下为Python的代码实现:
```python
import math
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
def distance_to(self, other_point):
"""
计算该点与另一个点之间的距离
"""
distance = math.sqrt((self.x - other_point.x) ** 2 + (self.y - other_point.y) ** 2)
return distance
```
这个类包含了一个初始化方法 `__init__()`,它会初始化点的坐标属性。还有一个计算距离的方法 `distance_to()`,它接收另一个点作为参数,计算两个点之间的距离并返回。其中,使用了Python内置的数学库`math`,调用了`sqrt()`函数来计算平方根。
### 回答2:
```python
import math
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
def calculate_distance(self, other):
distance = math.sqrt((self.x - other.x)**2 + (self.y - other.y)**2)
return distance
# 示例用法
point1 = Point(2, 3)
point2 = Point(5, 7)
distance = point1.calculate_distance(point2)
print(distance)
```
以上代码定义了一个名为`Point`的类,该类拥有属性`x`和`y`,分别表示点的坐标。还有一个名为`calculate_distance`的方法,用于计算两点之间的距离,参数为另一个点对象。
在示例用法中,分别创建了两个点`point1`和`point2`,并且调用`point1`的`calculate_distance`方法,传入`point2`作为参数来计算两点之间的距离。最后将计算结果打印出来。
这段代码中使用了数学库`math`中的`sqrt`函数来计算平方根,因此需要进行引入。
### 回答3:
以下是一个设计点的类的完整代码:
```python
import math
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
def distance(self, other_point):
dx = other_point.x - self.x
dy = other_point.y - self.y
distance = math.sqrt(dx**2 + dy**2)
return distance
```
在这段代码中,我们定义了一个名为Point的类。它有两个属性:x和y分别表示点的横坐标和纵坐标。此外,我们还为Point类定义了一个名为distance的方法。这个方法需要传入另一个点作为参数,然后计算当前点和传入点之间的距离。
在distance方法中,我们首先计算当前点和传入点在横坐标上的距离(dx),然后计算在纵坐标上的距离(dy)。最后,我们使用勾股定理计算两点之间的直线距离,并将其返回。
使用这个Point类,我们可以创建点的实例,并调用distance方法来计算两点之间的距离。下面是一个示例代码:
```python
# 创建两个点的实例
point1 = Point(0, 0)
point2 = Point(3, 4)
# 调用distance方法计算两点间的距离
distance = point1.distance(point2)
print(distance) # 输出结果为5.0
```
在这个示例代码中,我们创建了两个点的实例:一个点的坐标为(0, 0),另一个点的坐标为(3, 4)。然后,我们调用了point1的distance方法,将point2作为参数传入,计算了两点之间的距离。最后,我们将距离打印输出,结果为5.0。
阅读全文
相关推荐
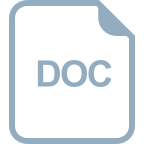
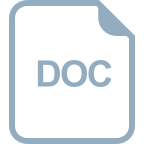













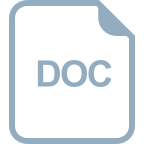
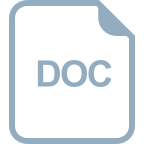
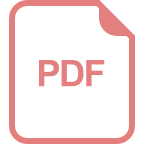