基于Python解析OPM文件实例
时间: 2024-04-09 17:11:48 浏览: 13
OPM文件是一种用于描述流程和业务过程的XML格式文件,通常用于业务流程管理和工作流程自动化。在Python中,可以使用xml.etree.ElementTree模块来解析OPM文件,以下是一个简单的示例。
假设我们有一个名为“example.opm”的OPM文件,它包含以下内容:
```
<?xml version="1.0" encoding="UTF-8"?>
<opm>
<process id="p1" name="Example Process">
<input name="input1" type="text" />
<output name="output1" type="text" />
<task id="t1" name="Task 1">
<input name="input2" type="text" />
<output name="output2" type="text" />
</task>
<task id="t2" name="Task 2">
<input name="input3" type="text" />
<output name="output3" type="text" />
</task>
<controlflow from="input1" to="t1.input2" />
<controlflow from="t1.output2" to="t2.input3" />
<controlflow from="t2.output3" to="output1" />
</process>
</opm>
```
我们可以使用以下代码解析该文件:
```python
import xml.etree.ElementTree as ET
# 打开OPM文件
tree = ET.parse('example.opm')
root = tree.getroot()
# 获取流程名称和ID
process_id = root[0].get('id')
process_name = root[0].get('name')
# 打印流程名称和ID
print('Process ID:', process_id)
print('Process Name:', process_name)
# 获取所有输入和输出
inputs = root.findall('.//input')
outputs = root.findall('.//output')
# 打印输入和输出
print('Inputs:')
for input in inputs:
print(' -', input.get('name'), '(', input.get('type'), ')')
print('Outputs:')
for output in outputs:
print(' -', output.get('name'), '(', output.get('type'), ')')
# 获取所有任务
tasks = root.findall('.//task')
# 打印任务名称和ID
print('Tasks:')
for task in tasks:
task_id = task.get('id')
task_name = task.get('name')
print(' Task ID:', task_id)
print(' Task Name:', task_name)
# 获取任务的输入和输出
inputs = task.findall('.//input')
outputs = task.findall('.//output')
# 打印任务的输入和输出
print(' Inputs:')
for input in inputs:
print(' -', input.get('name'), '(', input.get('type'), ')')
print(' Outputs:')
for output in outputs:
print(' -', output.get('name'), '(', output.get('type'), ')')
# 获取所有控制流
controlflows = root.findall('.//controlflow')
# 打印控制流
print('Control Flows:')
for controlflow in controlflows:
from_node = controlflow.get('from')
to_node = controlflow.get('to')
print(' -', from_node, '->', to_node)
```
运行以上代码,输出如下:
```
Process ID: p1
Process Name: Example Process
Inputs:
- input1 ( text )
Outputs:
- output1 ( text )
Tasks:
Task ID: t1
Task Name: Task 1
Inputs:
- input2 ( text )
Outputs:
- output2 ( text )
Task ID: t2
Task Name: Task 2
Inputs:
- input3 ( text )
Outputs:
- output3 ( text )
Control Flows:
- input1 -> t1.input2
- t1.output2 -> t2.input3
- t2.output3 -> output1
```
如上所示,我们成功地解析了OPM文件并提取了其中的流程、输入、输出、任务和控制流信息。
相关推荐
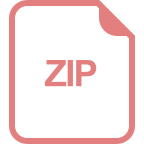
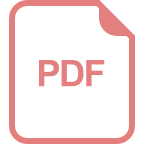














