pytorch用PINN求解间断初值条件下pde的代码
时间: 2023-06-30 10:04:09 浏览: 330
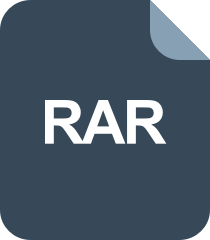
PDE算法代码

以下是使用 PyTorch 实现基于物理的神经网络 (PINN) 求解间断初值条件下的 PDE 的代码示例。
首先,我们需要导入必要的库:
```python
import torch
import torch.nn as nn
import torch.optim as optim
import numpy as np
import matplotlib.pyplot as plt
```
然后定义 PDE 的问题域和边界条件:
```python
def pde(x, t):
"""PDE function."""
return torch.exp(-t) * torch.sin(np.pi * x) + 0.5 * np.pi * torch.exp(-t) * torch.cos(np.pi * x) - torch.exp(-t) * np.pi**2 * torch.sin(np.pi * x)
def ic(x):
"""Initial condition."""
return torch.sin(np.pi * x)
def bc_l(t):
"""Left boundary condition."""
return 0.0
def bc_r(t):
"""Right boundary condition."""
return 0.0
```
接下来,我们定义神经网络模型:
```python
class PINN(nn.Module):
"""Physics-Informed Neural Network (PINN) model."""
def __init__(self, n_input, n_hidden, n_output):
super(PINN, self).__init__()
self.input_layer = nn.Linear(n_input, n_hidden)
self.hidden_layers = nn.ModuleList([nn.Linear(n_hidden, n_hidden) for _ in range(3)])
self.output_layer = nn.Linear(n_hidden, n_output)
def forward(self, x, t):
input_layer_output = torch.cat([x, t], dim=1)
hidden_layer_output = torch.tanh(self.input_layer(input_layer_output))
for hidden_layer in self.hidden_layers:
hidden_layer_output = torch.tanh(hidden_layer(hidden_layer_output))
output = self.output_layer(hidden_layer_output)
return output
```
然后,我们定义损失函数并进行训练:
```python
# Define input and output dimensions
n_input = 2
n_output = 1
# Define neural network model
model = PINN(n_input, 20, n_output)
# Define optimizer
optimizer = optim.Adam(model.parameters(), lr=0.001)
# Define loss function
def mse_loss(pred, target):
return torch.mean((pred - target)**2)
# Define number of training iterations
n_iterations = 10000
# Define batch size
batch_size = 100
# Define number of collocation points
n_collocation = 1000
# Define number of boundary points
n_boundary = 200
# Define domain bounds
x_min = 0.0
x_max = 1.0
t_min = 0.0
t_max = 1.0
# Generate training data
x_collocation = torch.rand(n_collocation, 1) * (x_max - x_min) + x_min
t_collocation = torch.rand(n_collocation, 1) * (t_max - t_min) + t_min
x_boundary_l = torch.zeros(n_boundary, 1) + x_min
t_boundary_l = torch.rand(n_boundary, 1) * (t_max - t_min) + t_min
x_boundary_r = torch.zeros(n_boundary, 1) + x_max
t_boundary_r = torch.rand(n_boundary, 1) * (t_max - t_min) + t_min
x_ic = torch.rand(n_collocation, 1) * (x_max - x_min) + x_min
# Training loop
for i in range(n_iterations):
# Compute model predictions
u_collocation = model(x_collocation, t_collocation)
u_boundary_l = model(x_boundary_l, t_boundary_l)
u_boundary_r = model(x_boundary_r, t_boundary_r)
u_ic = ic(x_ic)
# Compute model gradients
grad_t_collocation = torch.autograd.grad(u_collocation.sum(), t_collocation, create_graph=True)[0]
grad_x_collocation = torch.autograd.grad(u_collocation.sum(), x_collocation, create_graph=True)[0]
grad_x_boundary_l = torch.autograd.grad(u_boundary_l.sum(), x_boundary_l, create_graph=True)[0]
grad_x_boundary_r = torch.autograd.grad(u_boundary_r.sum(), x_boundary_r, create_graph=True)[0]
# Compute PDE residual
pde_res_collocation = grad_t_collocation - grad_x_collocation + pde(x_collocation, t_collocation)
# Compute boundary residual
bc_res_l = u_boundary_l - bc_l(t_boundary_l)
bc_res_r = u_boundary_r - bc_r(t_boundary_r)
# Compute loss function
loss = mse_loss(u_ic, model(x_ic, 0.0)) + mse_loss(pde_res_collocation, torch.zeros_like(pde_res_collocation)) + mse_loss(bc_res_l, torch.zeros_like(bc_res_l)) + mse_loss(bc_res_r, torch.zeros_like(bc_res_r))
# Backpropagation
optimizer.zero_grad()
loss.backward()
optimizer.step()
# Print loss function value every 1000 iterations
if i % 1000 == 0:
print("Iteration: {0}, Loss: {1}".format(i, loss.item()))
```
最后,我们可以使用训练好的模型绘制结果:
```python
# Generate test data
x_test = torch.linspace(x_min, x_max, 1000).reshape(-1, 1)
t_test = torch.linspace(t_min, t_max, 1000).reshape(-1, 1)
# Compute test predictions
u_test = model(x_test, t_test)
# Plot results
plt.figure()
plt.plot(x_test.detach().numpy(), u_test.detach().numpy(), label="PINN")
plt.xlabel("x")
plt.ylabel("u")
plt.legend()
plt.show()
```
这样,我们就完成了使用 PyTorch 实现基于物理的神经网络 (PINN) 求解间断初值条件下的 PDE 的代码。
阅读全文
相关推荐
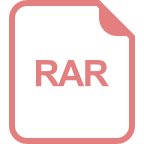
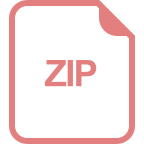




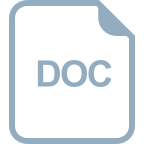


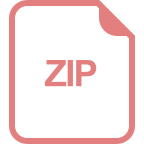
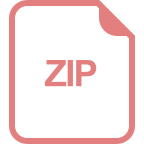
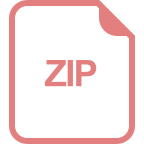
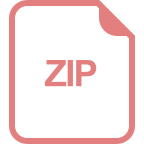