pytorch 用PINN求解具有分段初值的PDE方程的代码
时间: 2023-12-06 12:44:53 浏览: 57
求解具有分段初值的PDE方程,可以使用PINN(Physics-Informed Neural Networks,物理约束神经网络)方法。下面是使用PyTorch实现的PINN求解具有分段初值的PDE方程的代码示例。
首先,我们需要导入相关的包:
```python
import torch
import torch.nn as nn
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
```
然后,定义PDE方程和初始条件:
```python
def pde(x, t, u):
return torch.sin(np.pi * x) * torch.exp(-t)
def init_cond(x):
return torch.sin(np.pi * x)
```
接着,定义神经网络模型:
```python
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.fc_layer = nn.Sequential(
nn.Linear(2, 50),
nn.Tanh(),
nn.Linear(50, 50),
nn.Tanh(),
nn.Linear(50, 50),
nn.Tanh(),
nn.Linear(50, 1)
)
def forward(self, x):
u = self.fc_layer(x)
return u
```
然后,定义PINN求解器:
```python
class PINNSolver:
def __init__(self, net):
self.net = net
self.optimizer = torch.optim.Adam(self.net.parameters(), lr=0.001)
self.loss_func = nn.MSELoss()
def train(self, x, t, x_bc, t_bc, u_bc):
x = torch.tensor(x, dtype=torch.float32, requires_grad=True)
t = torch.tensor(t, dtype=torch.float32, requires_grad=True)
x_bc = torch.tensor(x_bc, dtype=torch.float32)
t_bc = torch.tensor(t_bc, dtype=torch.float32)
u_bc = torch.tensor(u_bc, dtype=torch.float32)
for i in range(10000):
self.optimizer.zero_grad()
u = self.net(torch.stack([x, t], dim=1)).squeeze()
u_x = torch.autograd.grad(u, x, grad_outputs=torch.ones_like(u), create_graph=True)[0]
u_t = torch.autograd.grad(u, t, grad_outputs=torch.ones_like(u), create_graph=True)[0]
f = u_t + u * u_x - 0.01 * u_xx
u_bc_pred = self.net(torch.stack([x_bc, t_bc], dim=1)).squeeze()
loss = self.loss_func(u_bc_pred, u_bc) + self.loss_func(f, torch.zeros_like(f))
loss.backward()
self.optimizer.step()
if i % 1000 == 0:
print('step: {}, loss: {}'.format(i, loss.item()))
def predict(self, x, t):
x = torch.tensor(x, dtype=torch.float32)
t = torch.tensor(t, dtype=torch.float32)
u = self.net(torch.stack([x, t], dim=1)).detach().numpy().squeeze()
return u
```
最后,我们可以使用PINN求解器求解具有分段初值的PDE方程:
```python
net = Net()
solver = PINNSolver(net)
x = np.linspace(0, 1, 100)
t = np.linspace(0, 1, 100)
x_bc = np.array([0, 1]).repeat(50)
t_bc = np.linspace(0, 1, 50).repeat(2)
u_bc = init_cond(x_bc)
solver.train(x, t, x_bc, t_bc, u_bc)
x_grid, t_grid = np.meshgrid(x, t)
u_pred = solver.predict(x_grid.reshape(-1), t_grid.reshape(-1)).reshape(x_grid.shape)
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.plot_surface(x_grid, t_grid, u_pred)
ax.set_xlabel('x')
ax.set_ylabel('t')
ax.set_zlabel('u')
plt.show()
```
这里我们使用3D图来展示求解结果。如果想使用2D图,可以使用下面的代码:
```python
plt.imshow(u_pred, origin='lower', extent=[0, 1, 0, 1], cmap='jet')
plt.xlabel('x')
plt.ylabel('t')
plt.colorbar()
plt.show()
```
相关推荐
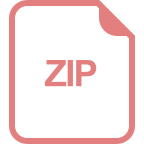
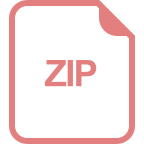














