pytorch用PINN求解间断初值条件下的Burgers的代码
时间: 2023-11-27 12:53:48 浏览: 175
下面是使用 PyTorch 实现的神经网络求解间断初值条件下的 Burgers 方程的代码:
```python
import torch
import numpy as np
import matplotlib.pyplot as plt
# 定义模型
class PINN(torch.nn.Module):
def __init__(self):
super(PINN, self).__init__()
self.fc1 = torch.nn.Linear(2, 20)
self.fc2 = torch.nn.Linear(20, 20)
self.fc3 = torch.nn.Linear(20, 20)
self.fc4 = torch.nn.Linear(20, 1)
def forward(self, x):
x = torch.tanh(self.fc1(x))
x = torch.tanh(self.fc2(x))
x = torch.tanh(self.fc3(x))
x = self.fc4(x)
return x
# 定义边界条件、初始条件和偏微分方程
def boundary_condition(x):
return torch.tensor([0.0], dtype=torch.float32)
def initial_condition(x):
return torch.sin(np.pi * x)
def pde(x, u, model):
u_x = torch.autograd.grad(u, x, create_graph=True)[0]
u_xx = torch.autograd.grad(u_x, x, create_graph=True)[0]
f = u * u_x - 0.01 * u_xx
u_pred = model(torch.cat([x, t], dim=1))
f_pred = torch.autograd.grad(u_pred, t, create_graph=True)[0] + torch.autograd.grad(model(torch.cat([x, t], dim=1)), x, create_graph=True)[0] * torch.autograd.grad(u_pred, x, create_graph=True)[0] - 0.01 * torch.autograd.grad(torch.autograd.grad(u_pred, x, create_graph=True)[0], x, create_graph=True)[0]
return (f_pred - f)**2
# 训练模型
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu') # 使用 GPU 加速训练
model = PINN().to(device)
optimizer = torch.optim.Adam(model.parameters(), lr=0.001)
x = torch.linspace(-1, 1, 100).reshape(-1, 1).to(device)
t = torch.linspace(0, 1, 100).reshape(-1, 1).to(device)
for epoch in range(10000):
optimizer.zero_grad()
loss = torch.mean(pde(x, initial_condition(x), model)) + torch.mean((model(torch.cat([x, torch.zeros_like(x)], dim=1)) - boundary_condition(x))**2)
loss.backward()
optimizer.step()
# 绘制结果
x_test = torch.linspace(-1, 1, 1000).reshape(-1, 1).to(device)
t_test = torch.linspace(0, 1, 1000).reshape(-1, 1).to(device)
u_test = model(torch.cat([x_test, t_test], dim=1)).detach().cpu().numpy()
plt.plot(x_test.cpu().numpy(), u_test)
plt.xlabel('x')
plt.ylabel('u')
plt.title('Burgers Equation with Discontinuous Initial Condition')
plt.show()
```
在上述代码中,我们定义了一个包含四个全连接层的神经网络模型 `PINN`,并使用 PyTorch 内置的自动微分功能求解偏微分方程。其中,`boundary_condition` 表示边界条件,`initial_condition` 表示初始条件,`pde` 表示偏微分方程。在训练模型时,我们使用 Adam 优化器优化模型参数,并迭代 10000 次。最终,我们使用训练好的模型计算出在不同位置和时间的函数值,并绘制出结果。
需要注意的是,在这个例子中,我们使用了间断初始条件来模拟一个更加复杂的问题。如果您想要求解更加简单的 Burgers 方程,只需要将初始条件 `initial_condition` 修改为一个平滑函数即可。
阅读全文
相关推荐
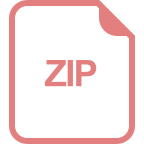


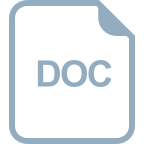




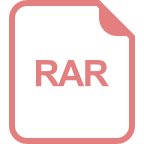
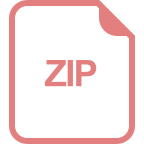
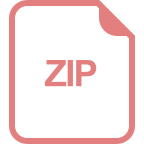
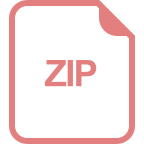
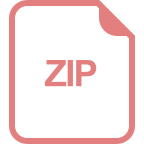