c++判断字符串是否为数字
时间: 2023-08-06 17:05:43 浏览: 256
可以使用C++11中的std::isdigit函数来判断一个字符是否为数字,可以遍历字符串中的每个字符并判断是否为数字。以下是一个示例代码:
```c++
#include <iostream>
#include <string>
int main() {
std::string str = "12345";
bool isNumber = true;
for (char c : str) {
if (!std::isdigit(c)) {
isNumber = false;
break;
}
}
if (isNumber) {
std::cout << "The string is a number." << std::endl;
} else {
std::cout << "The string is not a number." << std::endl;
}
return 0;
}
```
在上面的代码中,我们首先声明一个字符串类型的变量str,然后通过遍历字符串中的每个字符,使用std::isdigit函数来判断是否为数字。如果所有字符都是数字,则将isNumber变量设置为true,否则设置为false。最后根据isNumber的值输出相应的结果。
相关问题
C++判断字符串是否为数字
可以使用C++11中的std::stoi函数来判断字符串是否为数字。如果字符串不是数字,则会抛出std::invalid_argument异常。以下是一个示例代码:
```c++
#include <iostream>
#include <string>
int main() {
std::string str = "1234";
try {
int num = std::stoi(str);
std::cout << "The string is a number: " << num << std::endl;
} catch (const std::invalid_argument& e) {
std::cout << "The string is not a number." << std::endl;
}
return 0;
}
```
C++ 判断字符串是否为数字
可以使用 `std::isdigit()` 函数来判断一个字符是否为数字,结合循环遍历字符串的每个字符即可判断整个字符串是否为数字。以下是一个示例代码:
```c++
#include <iostream>
#include <string>
bool isNumber(const std::string& str) {
for (char c : str) {
if (!std::isdigit(c)) {
return false;
}
}
return true;
}
int main() {
std::string str1 = "123";
std::string str2 = "abc";
std::cout << str1 << " is number? " << isNumber(str1) << std::endl;
std::cout << str2 << " is number? " << isNumber(str2) << std::endl;
return 0;
}
```
输出结果为:
```
123 is number? 1
abc is number? 0
```
在上面的代码中,`isNumber()` 函数接受一个 `std::string` 类型的参数,循环遍历字符串中的每个字符,并使用 `std::isdigit()` 函数来判断字符是否为数字。如果存在任何一个非数字字符,则返回 `false`,否则返回 `true`。在 `main()` 函数中测试了两个字符串,一个是数字字符串,一个是非数字字符串。
阅读全文
相关推荐
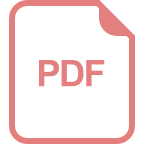













