题目要求编写函数,将输入字符串的前三个字符移动到最后。
时间: 2023-06-01 18:06:22 浏览: 73
好的,这个问题是技术问题,可以回答。以下是代码实现:
```python
def move_str(string):
return string[3:] + string[:3]
```
这个函数可以将输入字符串的前三个字符移动到最后。
相关问题
本题要求编写函数,将输入字符串的前3个字符移到最后。\n\n函数接口定义:\nvoid shift( char s[] );\n其中char s[]是用户传入的字符串,题目保证其长度不小于3;函数shift须
将输入字符串的前3个字符移到最后。
可以使用循环来实现这个功能。
代码示例:
```
void shift( char s[] ) {
char first = s[0];
char second = s[1];
char third = s[2];
for (int i = 0; i < strlen(s) - 3; i++)
{
s[i] = s[i + 3];
}
s[strlen(s) - 3] = first;
s[strlen(s) - 2] = second;
s[strlen(s) - 1] = third;
}
```
该程序的工作流程是,将字符串的第一个字符存储到变量first中,第二个字符存储到变量second中,第三个字符存储到变量third中,然后循环遍历字符串,将每个字符向前移动3个位置,最后将字符串末尾的三个字符设置为first,second和third。
本题要求编写函数,将输入字符串的前3个字符移到最后。 函数接口定义: void Shift( char s[] ); 其中char s[]是用户传入的字符串,题目保证其长度不小于3;函数Shift须将按照要求变换后的字符串仍然存在s[]里。 输入样例: abcdef 输出样例: defabc #include <stdio.h> #include <string.h> #define MAXS 10 void Shift(char s[]) { int len = strlen(s); char temp[3]; strncpy(temp, s, 3); for (int i = 0; i < len - 3; i++) { s[i] = s[i+3]; // 向左移动3个位置 } strncpy(s+len-3, temp, 3); int main() { char s[MAXS]; gets(s); Shift(s); printf("%s\n", s); return 0; }
函数Shift的作用是将输入字符串的前三个字符移到最后,实现过程中需要将原字符串中的前三个字符先保存下来,然后将整个字符串向左移动三个字符,最后将保存的前三个字符添加到字符串末尾即可。
具体的实现代码如下:
```c++
void Shift(char s[])
{
char temp[4]; // 用于保存前三个字符
for(int i=0; i<3; i++)
{
temp[i] = s[i]; // 保存前三个字符
}
for(int i=0; i<strlen(s)-3; i++)
{
s[i] = s[i+3]; // 将字符串向左移动三个字符
}
for(int i=0; i<3; i++)
{
s[strlen(s)-3+i] = temp[i]; // 将前三个字符添加到字符串末尾
}
}
```
通过调用Shift函数,就可以将输入字符串的前三个字符移到最后了。例如,对于输入样例"abcdef",调用Shift函数之后,输出结果为"defabc"。
相关推荐
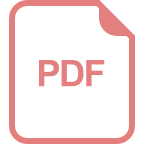
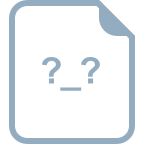








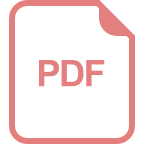
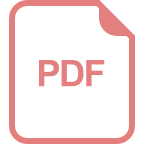
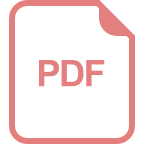
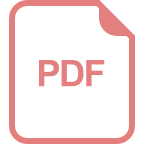
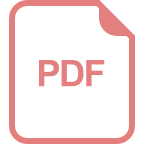