from multiprocessing import Process def f(name): print('hello', name) if __name__ == '__main__': p = Process(target=f, args=('bob',)) p.start() p.join()
时间: 2023-11-20 18:07:19 浏览: 28
这是一个使用 Python 的 multiprocessing 模块创建进程的代码示例。在这个例子中,首先定义了一个函数 f,它接受一个参数 name,打印出 "hello" 和 name。然后,在主程序中创建了一个 Process 对象 p,它的 target 参数指定为函数 f,args 参数为一个元组 ('bob',)。接着,通过调用 p.start() 方法启动进程,然后调用 p.join() 方法等待该进程结束。在进程执行过程中,会输出 "hello bob"。
相关问题
if __name__ == "__main__": freeze_support()python运行时添加,什么意思
`if __name__ == "__main__":`是Python中一个常用的条件语句,它的作用是判断当前模块是否是主程序入口。当一个Python文件被直接运行时,其`__name__`属性的值为`__main__`,而当它被作为模块导入时,`__name__`属性的值为模块名。因此,通过判断`__name__`属性是否等于`__main__`,可以确定当前模块是否是主程序入口。
在上述代码中,`freeze_support()`是`multiprocessing`模块中的一个函数,它的作用是为了在Windows平台下使用`multiprocessing`模块时避免出现一些问题。具体来说,它会在Windows平台下开启一个新的进程,并将该进程的执行入口设置为当前模块的主程序入口。这样做的目的是为了避免在Windows平台下出现一些由于多进程导致的问题,例如进程无法正常退出等。
因此,当我们在Windows平台下使用`multiprocessing`模块时,需要在程序的入口处添加`if __name__ == "__main__":`条件语句,并在其中调用`freeze_support()`函数,以确保程序能够正常运行。
代码示例:
```python
import multiprocessing
def func():
print("hello world")
if __name__ == "__main__":
multiprocessing.freeze_support()
p = multiprocessing.Process(target=func)
p.start()
p.join()
```
import multiprocessing as mp def foo(q): q.put('hello') if __name__ == '__main__': mp.set_start_method('spawn') q = mp.Queue() p = mp.Process(target=foo, args=(q,)) p.start() print(q.get()) p.join()
这段代码是一个使用 multiprocessing 模块实现的进程间通信的例子。它创建了一个进程 p,将一个 Queue 对象 q 作为参数传递给进程 p。进程 p 执行函数 foo,将字符串 'hello' 放入 Queue 对象 q 中。主进程通过调用 q.get() 从 Queue 对象 q 中取出了 'hello' 字符串并打印,最后通过 p.join() 等待进程 p 结束。该进程间通信方式是使用 Queue 对象实现的,由于 Queue 对象是线程安全的,因此可以在多个进程中安全地使用。这里使用了 spawn 的启动方式,因为它比 fork 更适合在 Windows 平台上使用。
相关推荐
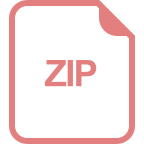
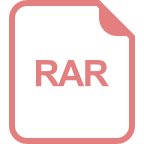
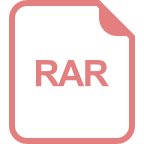












