标准c语言实现cgi通讯录
时间: 2023-07-13 19:07:21 浏览: 121
首先,需要了解CGI是什么。CGI是一种通用网关接口,它允许Web服务器运行外部程序,并将其输出返回给Web浏览器。在实现CGI通讯录时,需要编写一个C程序作为CGI程序,该程序将实现通讯录的添加、删除、修改和查询等功能。
以下是一个简单的CGI通讯录示例程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_NAME_LEN 50
#define MAX_PHONE_LEN 20
#define MAX_EMAIL_LEN 50
struct Contact {
char name[MAX_NAME_LEN + 1];
char phone[MAX_PHONE_LEN + 1];
char email[MAX_EMAIL_LEN + 1];
};
void add_contact(struct Contact *contacts, int *count, char *name, char *phone, char *email) {
if (*count >= 100) {
printf("Too many contacts!\n");
return;
}
struct Contact *contact = &contacts[*count];
strncpy(contact->name, name, MAX_NAME_LEN);
strncpy(contact->phone, phone, MAX_PHONE_LEN);
strncpy(contact->email, email, MAX_EMAIL_LEN);
(*count)++;
printf("Contact added successfully!\n");
}
void delete_contact(struct Contact *contacts, int *count, char *name) {
int index = -1;
for (int i = 0; i < *count; i++) {
if (strcmp(contacts[i].name, name) == 0) {
index = i;
break;
}
}
if (index == -1) {
printf("Contact not found!\n");
return;
}
for (int i = index; i < *count - 1; i++) {
contacts[i] = contacts[i + 1];
}
(*count)--;
printf("Contact deleted successfully!\n");
}
void modify_contact(struct Contact *contacts, int *count, char *name, char *phone, char *email) {
int index = -1;
for (int i = 0; i < *count; i++) {
if (strcmp(contacts[i].name, name) == 0) {
index = i;
break;
}
}
if (index == -1) {
printf("Contact not found!\n");
return;
}
struct Contact *contact = &contacts[index];
strncpy(contact->phone, phone, MAX_PHONE_LEN);
strncpy(contact->email, email, MAX_EMAIL_LEN);
printf("Contact modified successfully!\n");
}
void query_contact(struct Contact *contacts, int count, char *name) {
int index = -1;
for (int i = 0; i < count; i++) {
if (strcmp(contacts[i].name, name) == 0) {
index = i;
break;
}
}
if (index == -1) {
printf("Contact not found!\n");
return;
}
struct Contact *contact = &contacts[index];
printf("Name: %s\nPhone: %s\nEmail: %s\n", contact->name, contact->phone, contact->email);
}
int main(int argc, char *argv[]) {
struct Contact contacts[100];
int count = 0;
char *method = getenv("REQUEST_METHOD");
if (method == NULL) {
printf("Invalid request!\n");
return 1;
}
char *query_string = getenv("QUERY_STRING");
if (query_string == NULL) {
printf("Invalid request!\n");
return 1;
}
if (strcmp(method, "GET") == 0) {
char *name = strtok(query_string, "&=");
char *value = strtok(NULL, "&=");
char *phone = NULL, *email = NULL;
while (name != NULL && value != NULL) {
if (strcmp(name, "name") == 0) {
value = strtok(NULL, "&=");
strncpy(contacts[count].name, value, MAX_NAME_LEN);
} else if (strcmp(name, "phone") == 0) {
phone = value;
} else if (strcmp(name, "email") == 0) {
email = value;
}
name = strtok(NULL, "&=");
value = strtok(NULL, "&=");
}
if (phone != NULL && email != NULL) {
strncpy(contacts[count].phone, phone, MAX_PHONE_LEN);
strncpy(contacts[count].email, email, MAX_EMAIL_LEN);
count++;
printf("Contact added successfully!\n");
} else if (phone != NULL) {
query_contact(contacts, count, contacts[count - 1].name);
} else {
printf("Invalid request!\n");
}
} else if (strcmp(method, "POST") == 0) {
char *content_type = getenv("CONTENT_TYPE");
if (content_type == NULL || strcmp(content_type, "application/x-www-form-urlencoded") != 0) {
printf("Invalid request!\n");
return 1;
}
char *content_length_str = getenv("CONTENT_LENGTH");
if (content_length_str == NULL) {
printf("Invalid request!\n");
return 1;
}
int content_length = atoi(content_length_str);
char *post_data = (char *) malloc(content_length + 1);
if (post_data == NULL) {
printf("Out of memory!\n");
return 1;
}
fgets(post_data, content_length + 1, stdin);
char *name = strtok(post_data, "&=");
char *value = strtok(NULL, "&=");
char *phone = NULL, *email = NULL;
while (name != NULL && value != NULL) {
if (strcmp(name, "name") == 0) {
value = strtok(NULL, "&=");
strncpy(contacts[count].name, value, MAX_NAME_LEN);
} else if (strcmp(name, "phone") == 0) {
phone = value;
} else if (strcmp(name, "email") == 0) {
email = value;
}
name = strtok(NULL, "&=");
value = strtok(NULL, "&=");
}
if (phone != NULL && email != NULL) {
strncpy(contacts[count].phone, phone, MAX_PHONE_LEN);
strncpy(contacts[count].email, email, MAX_EMAIL_LEN);
count++;
printf("Contact added successfully!\n");
} else if (phone != NULL) {
query_contact(contacts, count, contacts[count - 1].name);
} else {
printf("Invalid request!\n");
}
free(post_data);
} else {
printf("Invalid request!\n");
return 1;
}
return 0;
}
```
该程序使用了标准C语言,实现了添加、删除、修改和查询通讯录的功能。在GET请求中,通过解析QUERY_STRING参数来获取参数值。在POST请求中,通过解析POST数据来获取参数值。其中,使用了CGI环境变量来获取请求方法、请求参数等信息。
该程序实现了一个简单的通讯录功能,但是由于没有进行输入验证和安全检查,存在安全隐患,建议在生产环境中使用时进行安全加固。
阅读全文
相关推荐



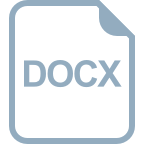

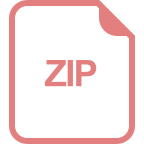

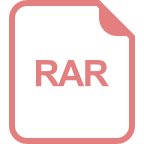
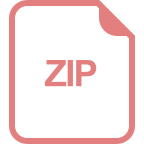
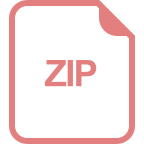
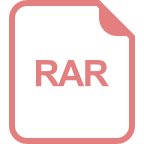