使用cpp使用BFS搜索算法从左上开始遍历,使用最小遍历次数找到最近不为-1的程序
时间: 2024-06-09 21:06:41 浏览: 88
以下是使用 C++ 编写的 BFS 搜索算法,用于从左上角开始遍历矩阵,找到最近的不为 -1 的数字。假设矩阵大小为 n x n,矩阵中的元素为整数,-1 表示该位置不可达。
```cpp
#include <iostream>
#include <queue>
#include <vector>
using namespace std;
const int MAXN = 1000;
int n;
int maze[MAXN][MAXN];
bool visited[MAXN][MAXN];
int dist[MAXN][MAXN];
vector<pair<int, int>> dirs = {{-1, 0}, {0, -1}, {1, 0}, {0, 1}};
bool isValid(int x, int y) {
return x >= 0 && x < n && y >= 0 && y < n && maze[x][y] != -1 && !visited[x][y];
}
int bfs(int startX, int startY) {
queue<pair<int, int>> q;
q.push({startX, startY});
visited[startX][startY] = true;
dist[startX][startY] = 0;
while (!q.empty()) {
auto curr = q.front();
q.pop();
for (auto& dir : dirs) {
int nx = curr.first + dir.first;
int ny = curr.second + dir.second;
if (isValid(nx, ny)) {
visited[nx][ny] = true;
dist[nx][ny] = dist[curr.first][curr.second] + 1;
if (maze[nx][ny] != -1) { // 找到第一个不为 -1 的数字
return dist[nx][ny];
}
q.push({nx, ny});
}
}
}
return -1; // 找不到不为 -1 的数字
}
int main() {
cin >> n;
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
cin >> maze[i][j];
}
}
int ans = bfs(0, 0);
cout << ans << endl;
return 0;
}
```
在上述代码中,我们使用一个 `visited` 数组记录每个位置是否被访问过,使用一个 `dist` 数组记录从起点到该位置的最短距离。我们在队列中使用一个 `pair` 记录位置坐标,每次从队列中取出一个位置,然后遍历其四个方向,将未访问的、可达的位置加入队列中,同时更新它们的 `visited` 和 `dist` 数组。当遍历到的位置不为 -1 时,我们就找到了最近的不为 -1 的数字,返回该位置的 `dist` 值即可。如果整个矩阵中没有不为 -1 的数字,就返回 -1。
阅读全文
相关推荐
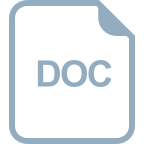
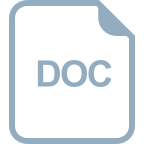
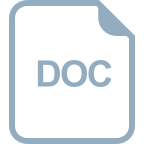

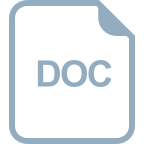
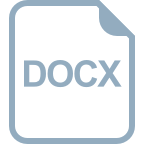
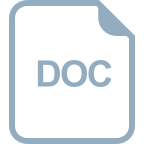
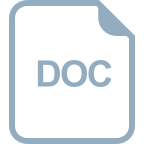
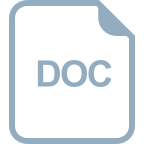
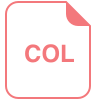
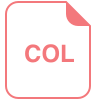



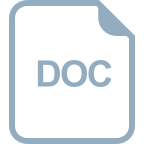
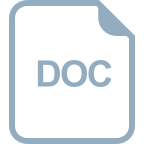
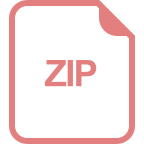
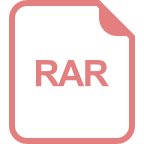
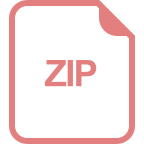