Linux文件的读写、定位方法代码步骤
时间: 2024-03-14 10:50:00 浏览: 56
读取文件的步骤如下:
1. 打开文件:使用open()函数打开文件,返回文件描述符。
2. 读取文件:使用read()函数从文件中读取数据,将数据存储在缓冲区中。
3. 关闭文件:使用close()函数关闭文件。
写入文件的步骤如下:
1. 打开文件:使用open()函数打开文件,返回文件描述符。
2. 写入文件:使用write()函数将数据写入文件。
3. 关闭文件:使用close()函数关闭文件。
定位文件的步骤如下:
1. 打开文件:使用open()函数打开文件,返回文件描述符。
2. 使用lseek()函数设置文件指针的位置。
3. 读取或写入文件:使用read()或write()函数读取或写入文件。
4. 关闭文件:使用close()函数关闭文件。
以下是读取文件的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
int main() {
int fd = open("test.txt", O_RDONLY); // 打开文件
if (fd < 0) {
perror("open");
exit(1);
}
char buf[1024];
int n = read(fd, buf, sizeof(buf)); // 读取文件
if (n < 0) {
perror("read");
exit(1);
}
printf("Read %d bytes: %s\n", n, buf);
close(fd); // 关闭文件
return 0;
}
```
以下是写入文件的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
int main() {
int fd = open("test.txt", O_WRONLY | O_CREAT, 0644); // 打开文件
if (fd < 0) {
perror("open");
exit(1);
}
char *str = "Hello, World!";
int n = write(fd, str, strlen(str)); // 写入文件
if (n < 0) {
perror("write");
exit(1);
}
printf("Write %d bytes: %s\n", n, str);
close(fd); // 关闭文件
return 0;
}
```
以下是定位文件的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
int main() {
int fd = open("test.txt", O_RDWR); // 打开文件
if (fd < 0) {
perror("open");
exit(1);
}
char buf[1024];
lseek(fd, 6, SEEK_SET); // 定位到文件的第7个字符
int n = read(fd, buf, sizeof(buf)); // 读取文件
if (n < 0) {
perror("read");
exit(1);
}
printf("Read %d bytes: %s\n", n, buf);
close(fd); // 关闭文件
return 0;
}
```
阅读全文
相关推荐
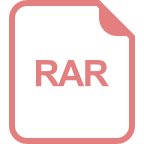
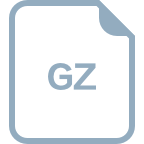
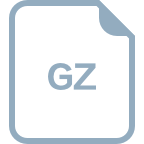
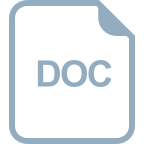
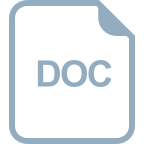
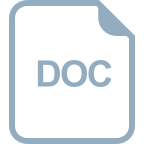
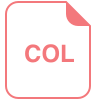
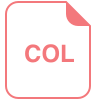
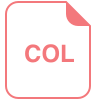
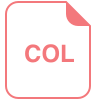
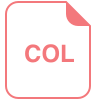
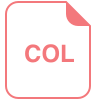
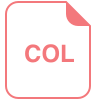
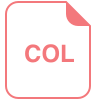
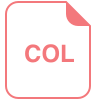
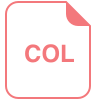
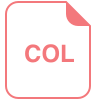