Write a program to complete the following command: new id - create a specified sequence of Numbers for id (id < 200000) Add id num - to join the sequence of Numbers for id integer num merge id1 id2 - merger sequence number of id1 and id2, and make id2 empty unique id - remove repetitive element in the sequence id out id - from small to large, output the elements of sequence of Numbers for ids separated by Spaces Input The First Line is n which mean the number of the Command (n <= 200000). The next n lines are correct Command. You don't have to worry wrong input and id is int. Output Then output according the requirements. Sample Input 16 new 1 new 2 add 1 1 add 1 2 add 1 3 add 2 1 add 2 2 add 2 3 add 2 4 out 1 out 2 merge 1 2 out 1 out 2 unique 1 out 1 16 new 1 new 2 add 1 1 add 1 2 add 1 3 add 2 1 add 2 2 add 2 3 add 2 4 out 1 out 2 merge 1 2 out 1 out 2 unique 1 out 1 Sample Ouput 1 2 3 1 2 3 4 1 1 2 2 3 3 4 1 2 3 4
时间: 2024-01-05 07:02:58 浏览: 27
以下是 Python3 的实现:
```python
from collections import defaultdict
def new(d, id):
if id not in d:
d[id] = []
def add(d, id, num):
d[id].append(num)
def merge(d, id1, id2):
d[id1] += d[id2]
d[id2] = []
def unique(d, id):
d[id] = list(set(d[id]))
def out(d, id):
print(' '.join(map(str, sorted(d[id]))))
n = int(input())
d = defaultdict(list)
for i in range(n):
args = input().split()
if args[0] == 'new':
new(d, int(args[1]))
elif args[0] == 'add':
add(d, int(args[1]), int(args[2]))
elif args[0] == 'merge':
merge(d, int(args[1]), int(args[2]))
elif args[0] == 'unique':
unique(d, int(args[1]))
elif args[0] == 'out':
out(d, int(args[1]))
```
思路是用 defaultdict 存储每个 id 对应的序列,然后根据命令逐个处理。代码中的 `new`、`add`、`merge`、`unique`、`out` 分别对应题目中的五个命令。
相关推荐
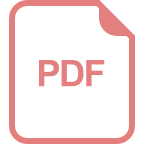
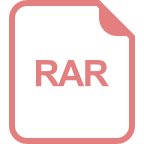
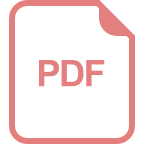




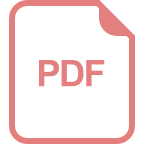
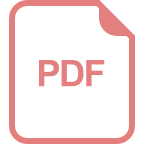
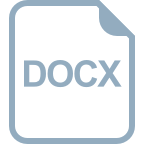
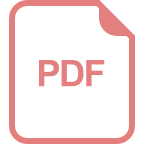
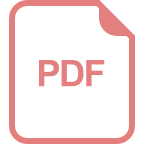
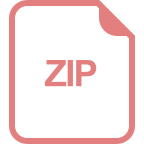
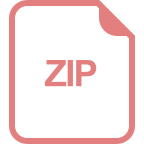
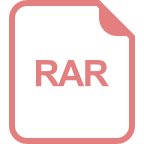
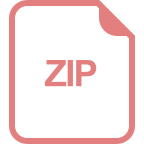