另一个进程从该管道中获取数据并写入文件(data.dat)
时间: 2023-08-07 20:05:18 浏览: 55
好的,我理解了你的问题。你可以通过以下步骤将管道中的数据写入文件data.dat:
1. 打开文件data.dat(如果文件不存在则创建它)。
2. 打开管道以从中读取数据。
3. 从管道中读取数据并将其写入data.dat文件中,直到管道中没有数据可读。
4. 关闭管道和文件。
以下是示例代码,演示如何将管道中的数据写入data.dat文件:
```python
import os
# 打开文件data.dat以写入数据
with open('data.dat', 'wb') as f:
# 打开管道以读取数据
pipe = os.open('mypipe', os.O_RDONLY)
while True:
# 从管道中读取数据
data = os.read(pipe, 1024)
if not data:
# 如果管道中没有数据可读,则退出循环
break
# 将数据写入文件data.dat中
f.write(data)
# 关闭管道和文件
os.close(pipe)
```
请注意,上述代码假定您已经创建了名为“mypipe”的管道,并且该管道已经被其他进程用于向它写入数据。如果管道不存在或没有数据可读,上述代码将退出循环并关闭管道和文件。
相关问题
一、一个进程输入一个学生信息(学号、姓名、成绩)并通过命名管道发送出去。另一个进程从该管道中获取数据并写入文件 (data.dat)。编写一个程序读取文件,检验结果。 二、编写通过共享内存实现的两个进程间按顺序通信
好的,我可以帮你解答这两个问题。
一、进程间通信,可以使用管道来实现。下面是一个简单的示例代码:
进程1:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/stat.h>
typedef struct {
int id;
char name[20];
float score;
} Student;
int main() {
int fd;
Student stu = { 101, "Tom", 89.5 };
// 创建命名管道
mkfifo("myfifo", 0666);
// 打开命名管道并写入数据
fd = open("myfifo", O_WRONLY);
write(fd, &stu, sizeof(Student));
close(fd);
return 0;
}
```
进程2:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/stat.h>
typedef struct {
int id;
char name[20];
float score;
} Student;
int main() {
int fd;
Student stu;
// 打开命名管道并读取数据
fd = open("myfifo", O_RDONLY);
read(fd, &stu, sizeof(Student));
close(fd);
// 将数据写入文件
FILE *fp = fopen("data.dat", "wb");
fwrite(&stu, sizeof(Student), 1, fp);
fclose(fp);
return 0;
}
```
进程3:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/stat.h>
typedef struct {
int id;
char name[20];
float score;
} Student;
int main() {
Student stu;
// 从文件中读取数据
FILE *fp = fopen("data.dat", "rb");
fread(&stu, sizeof(Student), 1, fp);
fclose(fp);
// 输出数据
printf("id: %d\nname: %s\nscore: %.1f\n", stu.id, stu.name, stu.score);
return 0;
}
```
这里使用了命名管道来实现进程间通信。进程1向管道中写入数据,进程2从管道中读取数据并写入文件,进程3从文件中读取数据并输出。
二、共享内存也可以用来实现进程间通信。下面是一个示例代码:
进程1:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/ipc.h>
#include <sys/shm.h>
typedef struct {
int id;
char name[20];
float score;
} Student;
int main() {
int shmid;
Student *stu;
key_t key = 1234;
// 创建共享内存
shmid = shmget(key, sizeof(Student), IPC_CREAT | 0666);
// 映射共享内存
stu = (Student*)shmat(shmid, NULL, 0);
// 写入数据
stu->id = 101;
strcpy(stu->name, "Tom");
stu->score = 89.5;
// 解除映射
shmdt(stu);
return 0;
}
```
进程2:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/ipc.h>
#include <sys/shm.h>
typedef struct {
int id;
char name[20];
float score;
} Student;
int main() {
int shmid;
Student *stu;
key_t key = 1234;
// 获取共享内存
shmid = shmget(key, sizeof(Student), 0666);
// 映射共享内存
stu = (Student*)shmat(shmid, NULL, 0);
// 读取数据并输出
printf("id: %d\nname: %s\nscore: %.1f\n", stu->id, stu->name, stu->score);
// 解除映射
shmdt(stu);
// 删除共享内存
shmctl(shmid, IPC_RMID, NULL);
return 0;
}
```
这里使用了共享内存来实现进程间通信。进程1将数据写入共享内存,进程2从共享内存中读取数据并输出。注意要在使用完共享内存后将其删除。
3. 编写程序,生成一个二进制数据文件,将n~m之间的所有素数写入文件data.dat中。从数据文件中读取二进制数据,并在显示器上以每行10个数的形式显示。
以下是Python实现的程序,可以生成一个二进制数据文件data.dat:
```python
import struct
def is_prime(n):
if n < 2:
return False
for i in range(2, int(n**0.5)+1):
if n % i == 0:
return False
return True
n = int(input("请输入起始数:"))
m = int(input("请输入结束数:"))
with open("data.dat", "wb") as f:
for i in range(n, m+1):
if is_prime(i):
f.write(struct.pack("i", i))
print("数据已生成并保存到data.dat中。")
```
接下来,我们可以编写另一个程序来读取这个二进制数据文件,并以每行10个数的形式显示:
```python
import struct
with open("data.dat", "rb") as f:
while True:
data = f.read(4)
if not data:
break
num = struct.unpack("i", data)[0]
print(num, end=" ")
if (num - n + 1) % 10 == 0:
print()
```
其中,`struct.pack("i", i)`将整数i打包成4个字节的二进制数据,写入文件中;`struct.unpack("i", data)`将从文件中读取的4个字节的二进制数据解析成一个整数。
相关推荐
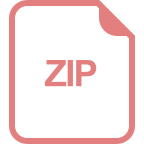
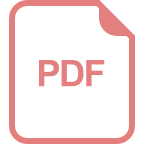
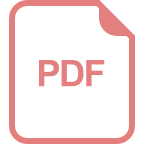








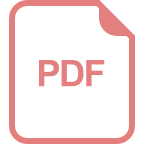