麻烦换成使用openssl库进行透明加密的代码示例
时间: 2023-10-24 20:14:46 浏览: 57
以下是一个使用 OpenSSL 库进行透明加密的示例代码,主要使用了 OpenSSL 的 BIO 接口:
```c
#include <openssl/err.h>
#include <openssl/evp.h>
#include <openssl/bio.h>
void handleErrors(void)
{
ERR_print_errors_fp(stderr);
abort();
}
int encrypt(unsigned char *plaintext, int plaintext_len, unsigned char *key,
unsigned char *iv, unsigned char *ciphertext)
{
EVP_CIPHER_CTX *ctx;
int len;
int ciphertext_len;
/* Create and initialise the context */
if (!(ctx = EVP_CIPHER_CTX_new())) {
handleErrors();
}
/* Initialise the encryption operation */
if (1 != EVP_EncryptInit_ex(ctx, EVP_aes_256_cbc(), NULL, key, iv)) {
handleErrors();
}
/* Provide the message to be encrypted, and obtain the encrypted output */
if (1 != EVP_EncryptUpdate(ctx, ciphertext, &len, plaintext, plaintext_len)) {
handleErrors();
}
ciphertext_len = len;
/* Finalise the encryption */
if (1 != EVP_EncryptFinal_ex(ctx, ciphertext + len, &len)) {
handleErrors();
}
ciphertext_len += len;
/* Clean up */
EVP_CIPHER_CTX_free(ctx);
return ciphertext_len;
}
int decrypt(unsigned char *ciphertext, int ciphertext_len, unsigned char *key,
unsigned char *iv, unsigned char *plaintext)
{
EVP_CIPHER_CTX *ctx;
int len;
int plaintext_len;
int ret;
/* Create and initialise the context */
if (!(ctx = EVP_CIPHER_CTX_new())) {
handleErrors();
}
/* Initialise the decryption operation */
if (1 != EVP_DecryptInit_ex(ctx, EVP_aes_256_cbc(), NULL, key, iv)) {
handleErrors();
}
/* Provide the message to be decrypted, and obtain the plaintext output */
if (1 != EVP_DecryptUpdate(ctx, plaintext, &len, ciphertext, ciphertext_len)) {
handleErrors();
}
plaintext_len = len;
/* Finalise the decryption */
ret = EVP_DecryptFinal_ex(ctx, plaintext + len, &len);
if (ret != 1) {
/* Clean up */
EVP_CIPHER_CTX_free(ctx);
return 0;
}
plaintext_len += len;
/* Clean up */
EVP_CIPHER_CTX_free(ctx);
return plaintext_len;
}
int encrypt_file(char *in_filename, char *out_filename, unsigned char *key,
unsigned char *iv)
{
EVP_CIPHER_CTX *ctx;
BIO *in, *out;
unsigned char inbuf[1024], outbuf[1024 + EVP_MAX_BLOCK_LENGTH];
int inlen, outlen, tmplen;
int ciphertext_len = 0;
/* Create and initialise the context */
if (!(ctx = EVP_CIPHER_CTX_new())) {
handleErrors();
}
/* Initialise the encryption operation */
if (1 != EVP_EncryptInit_ex(ctx, EVP_aes_256_cbc(), NULL, key, iv)) {
handleErrors();
}
/* Open the input and output files */
if ((in = BIO_new_file(in_filename, "rb")) == NULL) {
handleErrors();
}
if ((out = BIO_new_file(out_filename, "wb")) == NULL) {
handleErrors();
}
/* Process the input file */
while ((inlen = BIO_read(in, inbuf, 1024)) > 0) {
if (1 != EVP_EncryptUpdate(ctx, outbuf, &outlen, inbuf, inlen)) {
handleErrors();
}
if (BIO_write(out, outbuf, outlen) != outlen) {
handleErrors();
}
ciphertext_len += outlen;
}
/* Finalise the encryption */
if (1 != EVP_EncryptFinal_ex(ctx, outbuf, &tmplen)) {
handleErrors();
}
if (BIO_write(out, outbuf, tmplen) != tmplen) {
handleErrors();
}
ciphertext_len += tmplen;
/* Clean up */
BIO_free_all(in);
BIO_free_all(out);
EVP_CIPHER_CTX_free(ctx);
return ciphertext_len;
}
int decrypt_file(char *in_filename, char *out_filename, unsigned char *key,
unsigned char *iv)
{
EVP_CIPHER_CTX *ctx;
BIO *in, *out;
unsigned char inbuf[1024 + EVP_MAX_BLOCK_LENGTH], outbuf[1024];
int inlen, outlen, tmplen;
int plaintext_len = 0;
int ret;
/* Create and initialise the context */
if (!(ctx = EVP_CIPHER_CTX_new())) {
handleErrors();
}
/* Initialise the decryption operation */
if (1 != EVP_DecryptInit_ex(ctx, EVP_aes_256_cbc(), NULL, key, iv)) {
handleErrors();
}
/* Open the input and output files */
if ((in = BIO_new_file(in_filename, "rb")) == NULL) {
handleErrors();
}
if ((out = BIO_new_file(out_filename, "wb")) == NULL) {
handleErrors();
}
/* Process the input file */
while ((inlen = BIO_read(in, inbuf, 1024 + EVP_MAX_BLOCK_LENGTH)) > 0) {
ret = EVP_DecryptUpdate(ctx, outbuf, &outlen, inbuf, inlen);
if (ret != 1) {
/* Clean up */
BIO_free_all(in);
BIO_free_all(out);
EVP_CIPHER_CTX_free(ctx);
return 0;
}
if (BIO_write(out, outbuf, outlen) != outlen) {
handleErrors();
}
plaintext_len += outlen;
}
/* Finalise the decryption */
ret = EVP_DecryptFinal_ex(ctx, outbuf, &tmplen);
if (ret != 1) {
/* Clean up */
BIO_free_all(in);
BIO_free_all(out);
EVP_CIPHER_CTX_free(ctx);
return 0;
}
if (BIO_write(out, outbuf, tmplen) != tmplen) {
handleErrors();
}
plaintext_len += tmplen;
/* Clean up */
BIO_free_all(in);
BIO_free_all(out);
EVP_CIPHER_CTX_free(ctx);
return plaintext_len;
}
int main()
{
unsigned char key[EVP_MAX_KEY_LENGTH] = "01234567890123456789012345678901";
unsigned char iv[EVP_MAX_IV_LENGTH] = "0123456789012345";
char *in_filename = "input.txt";
char *out_filename = "output.txt";
int plaintext_len, ciphertext_len, decryptedtext_len;
/* Encrypt the input file */
ciphertext_len = encrypt_file(in_filename, out_filename, key, iv);
printf("Encrypted file size: %d bytes\n", ciphertext_len);
/* Decrypt the output file */
decryptedtext_len = decrypt_file(out_filename, "decrypted.txt", key, iv);
printf("Decrypted file size: %d bytes\n", decryptedtext_len);
return 0;
}
```
请注意,这只是一个示例代码,仅用于演示如何使用 OpenSSL 库进行透明加密。您需要根据自己的需求对代码进行修改。同时,为了确保安全性,请务必注意密钥的生成和存储,以及加密和解密的数据完整性。
相关推荐
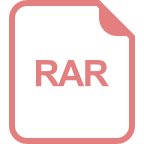
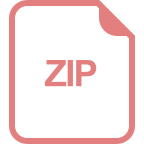
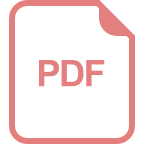














