java收取邮箱附件并提供邮箱附件的下载地址
时间: 2023-05-31 14:04:38 浏览: 210
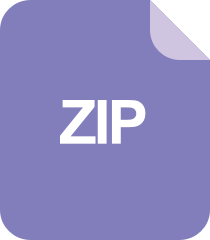
javamail收取邮件(包括附件)

以下是一个使用Java收取邮箱附件并提供下载地址的示例代码:
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.Properties;
import javax.mail.BodyPart;
import javax.mail.Flags;
import javax.mail.Folder;
import javax.mail.Message;
import javax.mail.Multipart;
import javax.mail.Part;
import javax.mail.Session;
import javax.mail.Store;
import javax.mail.internet.MimeBodyPart;
import javax.mail.internet.MimeMessage;
import javax.mail.internet.MimeMultipart;
public class EmailAttachmentDownloader {
public static void main(String[] args) {
String host = "imap.gmail.com";
String username = "your_email_address";
String password = "your_email_password";
String folderName = "INBOX";
String downloadFolder = "C:/Downloads";
Properties properties = new Properties();
properties.setProperty("mail.store.protocol", "imaps");
try {
Session session = Session.getInstance(properties);
Store store = session.getStore();
store.connect(host, username, password);
Folder folder = store.getFolder(folderName);
folder.open(Folder.READ_WRITE);
Message[] messages = folder.getMessages();
for (Message message : messages) {
if (message.getContentType().toLowerCase().startsWith("multipart/")) {
Multipart multipart = (Multipart) message.getContent();
for (int i = 0; i < multipart.getCount(); i++) {
BodyPart bodyPart = multipart.getBodyPart(i);
if (bodyPart.getDisposition() != null
&& bodyPart.getDisposition().equalsIgnoreCase(Part.ATTACHMENT)) {
String fileName = bodyPart.getFileName();
String filePath = downloadFolder + File.separator + fileName;
saveAttachment(bodyPart.getInputStream(), filePath);
System.out.println("Attachment downloaded: " + filePath);
}
}
}
message.setFlag(Flags.Flag.DELETED, true);
}
folder.close(true);
store.close();
} catch (Exception e) {
e.printStackTrace();
}
}
private static void saveAttachment(InputStream inputStream, String filePath) throws IOException {
FileOutputStream outputStream = new FileOutputStream(new File(filePath));
byte[] buffer = new byte[4096];
int bytesRead = -1;
while ((bytesRead = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
outputStream.close();
inputStream.close();
}
}
这个示例代码使用JavaMail API从IMAP服务器收取邮件,并遍历每个消息的所有附件。如果找到附件,它会将其保存到指定的本地文件夹中,并输出文件路径。最后,它将标记每个消息以删除。
你可以根据自己的需要修改这个代码来适应不同的邮件服务器和附件下载路径。
阅读全文
相关推荐
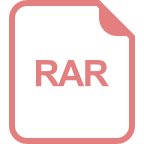
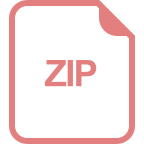
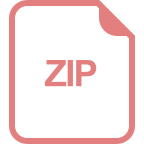
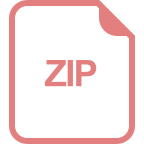
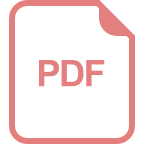
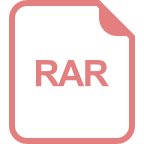
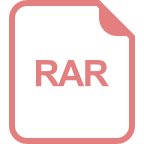
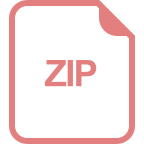
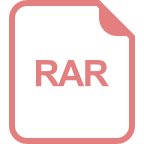
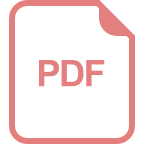
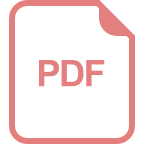
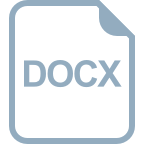
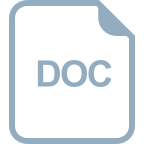
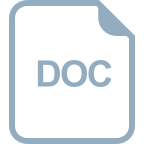
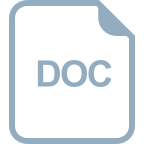
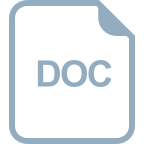