C++获取服务器性能指标-QPS 函数或者代码
时间: 2024-05-14 12:18:41 浏览: 201
获取服务器性能指标-QPS,可以使用以下代码:
```c++
#include <iostream>
#include <cstdio>
#include <chrono>
#include <thread>
#include <atomic>
#include <vector>
#include <string>
#include <algorithm>
#include <cstring>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <unistd.h>
void send_request(int sockfd) {
std::string request("GET / HTTP/1.0\r\n\r\n");
send(sockfd, request.c_str(), request.size(), 0);
}
void thread_func(const char* ip, int port, std::atomic<int>& count) {
int sockfd = socket(AF_INET, SOCK_STREAM, 0);
if (sockfd == -1) {
std::cout << "create socket failed\n";
return;
}
struct sockaddr_in serv_addr{};
memset(&serv_addr, 0, sizeof(serv_addr));
serv_addr.sin_family = AF_INET;
serv_addr.sin_addr.s_addr = inet_addr(ip);
serv_addr.sin_port = htons(port);
if (connect(sockfd, (struct sockaddr*)&serv_addr, sizeof(serv_addr)) == -1) {
std::cout << "connect failed\n";
return;
}
while (true) {
send_request(sockfd);
char buf[1024];
int len = recv(sockfd, buf, sizeof(buf), 0);
if (len == -1) {
std::cout << "recv failed\n";
break;
} else if (len == 0) {
std::cout << "server closed\n";
break;
}
count++;
}
close(sockfd);
}
int main() {
std::vector<std::thread> threads;
std::atomic<int> count{0};
const char* ip = "127.0.0.1";
int port = 80;
int thread_num = 10;
int seconds = 10;
for (int i = 0; i < thread_num; ++i) {
threads.emplace_back(thread_func, ip, port, std::ref(count));
}
std::this_thread::sleep_for(std::chrono::seconds(seconds));
for (auto& t : threads) {
t.join();
}
std::cout << "QPS: " << count / (double)seconds << '\n';
return 0;
}
```
该程序会创建多个线程,每个线程不断向服务器发送请求,并计算总共发送的请求数量,最后根据时间计算出 QPS 值。
需要注意的是,该程序只适用于 HTTP 协议的服务器,若要测试其他协议的服务器,需要修改发送请求的内容和解析响应的方式。同时,该程序也需要在 Linux 环境下编译运行。
阅读全文
相关推荐
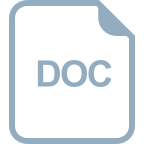
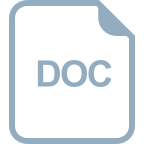
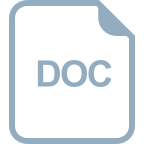



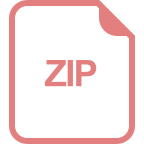
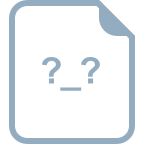
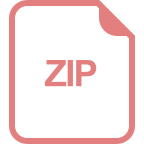
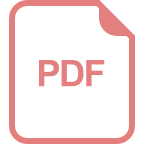
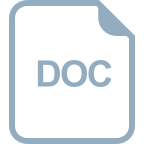
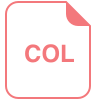
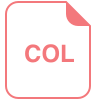
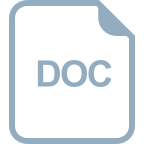
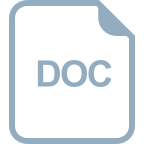
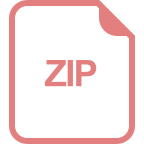
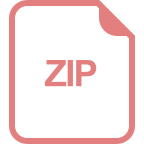
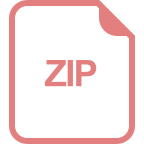
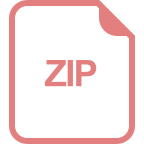