霍夫变换检测直线找到并画出其交点
时间: 2023-08-14 08:08:31 浏览: 57
霍夫变换可以用来检测图像中的直线。但是霍夫变换不能直接找到交点,需要进行一些额外的计算。
以下是基于OpenCV的Python代码,可以检测图像中的直线,并找到交点:
```python
import cv2
import numpy as np
# 读入图片
img = cv2.imread('image.jpg')
# 灰度化
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 边缘检测
edges = cv2.Canny(gray, 50, 150, apertureSize=3)
# 霍夫变换
lines = cv2.HoughLines(edges, 1, np.pi / 180, 200)
# 画出直线
for line in lines:
rho, theta = line[0]
a, b = np.cos(theta), np.sin(theta)
x0, y0 = a * rho, b * rho
pt1 = (int(x0 + 1000 * (-b)), int(y0 + 1000 * (a)))
pt2 = (int(x0 - 1000 * (-b)), int(y0 - 1000 * (a)))
cv2.line(img, pt1, pt2, (0, 0, 255), 3)
# 计算交点
for i in range(len(lines)):
for j in range(i + 1, len(lines)):
rho1, theta1 = lines[i][0]
rho2, theta2 = lines[j][0]
A = np.array([[np.cos(theta1), np.sin(theta1)], [np.cos(theta2), np.sin(theta2)]])
b = np.array([[rho1], [rho2]])
x0, y0 = np.linalg.solve(A, b)
x0, y0 = int(x0), int(y0)
cv2.circle(img, (x0, y0), 5, (0, 255, 0), -1)
# 显示结果
cv2.imshow('result', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
其中,`cv2.HoughLines` 函数用来进行霍夫变换,得到直线的参数。`cv2.line` 函数用来画出直线。计算交点使用了 numpy 的线性方程求解函数 `np.linalg.solve`。最后,使用 `cv2.circle` 函数画出交点。
相关推荐
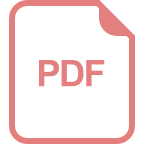
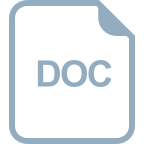
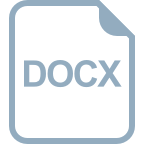
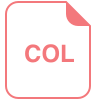
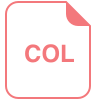
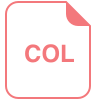
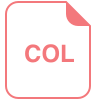










