1.定义一个数组,数组中包含产品的名称、单价和购买数量,使用methods(方法)computed(计算属性)和watch(侦听器)这3者中最优的方式计算购物车中产品的总价格;然后修改产品的购买数量,并重新计算其总价格。用vue写完整代码
时间: 2024-04-21 19:28:09 浏览: 128
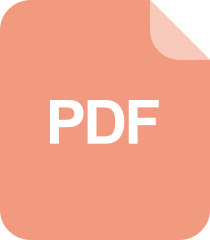
Vue.js计算机属性computed和methods方法详解

好的,下面是使用 Vue 完整实现购物车总价格计算的代码:
```vue
<template>
<div>
<ul>
<li v-for="(product, index) in products" :key="index">
{{ product.name }} - {{ product.price }} - {{ product.quantity }}
<button @click="incrementQuantity(product)">+</button>
<button @click="decrementQuantity(product)">-</button>
</li>
</ul>
<div>Total Price: {{ totalPrice }}</div>
</div>
</template>
<script>
export default {
data() {
return {
products: [
{ name: 'Product A', price: 10, quantity: 2 },
{ name: 'Product B', price: 5, quantity: 3 },
{ name: 'Product C', price: 8, quantity: 1 }
],
totalPrice: 0
};
},
mounted() {
this.calculateTotalPrice();
},
methods: {
incrementQuantity(product) {
product.quantity++;
},
decrementQuantity(product) {
if (product.quantity > 0) {
product.quantity--;
}
},
calculateTotalPrice() {
this.totalPrice = this.products.reduce((total, product) => {
return total + product.price * product.quantity;
}, 0);
}
},
computed: {
totalProducts() {
return this.products.reduce((total, product) => {
return total + product.quantity;
}, 0);
}
},
watch: {
products: {
handler() {
this.calculateTotalPrice();
},
deep: true
}
}
};
</script>
```
在上面的代码中,我们使用 `data` 方法来定义包含产品信息的数组 `products` 和总价格 `totalPrice`。在 `mounted` 钩子函数中,我们调用 `calculateTotalPrice` 方法来计算购物车中所有产品的总价格,并将其赋值给 `totalPrice`。
我们还定义了两个方法 `incrementQuantity` 和 `decrementQuantity`,用于增加和减少产品的数量。这两个方法会在模板中的 `button` 元素上绑定 `@click` 事件。
我们使用计算属性 `totalProducts` 来计算购物车中产品的总数量。这个计算属性会在模板中被使用。
最后,我们使用侦听器 `watch` 来监听 `products` 数组的变化,并在数组发生变化时重新计算总价格。
希望这个示例代码能够帮助到你!
阅读全文
相关推荐
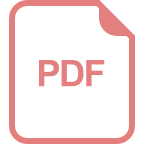
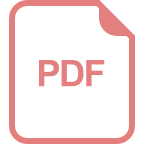















