设计简单几何工厂类,支持点、折线、圆形、矩形、三角形、多边线等几何形状。要求: (1)几何支持求面积、周长、判断是否包含给定点、几何类型、判断两个几何是否相交等 操作; (2)支持下列简单几何文本格式: Circle centerx entery radius Rectangle xmin ymin xmax ymax Triangle ax ay bx by cx cy
时间: 2023-05-11 22:02:51 浏览: 118
我可以为您提供一个简单的几何工厂类的设计,如下所示:
class GeometryFactory:
def create_geometry(self, geometry_type, *args):
if geometry_type == "point":
return Point(*args)
elif geometry_type == "line":
return Line(*args)
elif geometry_type == "circle":
return Circle(*args)
elif geometry_type == "rectangle":
return Rectangle(*args)
elif geometry_type == "triangle":
return Triangle(*args)
elif geometry_type == "polygon":
return Polygon(*args)
else:
raise ValueError("Invalid geometry type")
其中,Point、Line、Circle、Rectangle、Triangle 和 Polygon 都是几何形状的类,它们都实现了求面积、周长、判断是否包含给定点、几何类型、判断两个几何是否相交等操作。例如,Circle 类的实现如下:
class Circle:
def __init__(self, center_x, center_y, radius):
self.center_x = center_x
self.center_y = center_y
self.radius = radius
def area(self):
return math.pi * self.radius ** 2
def perimeter(self):
return 2 * math.pi * self.radius
def contains(self, point):
return (point.x - self.center_x) ** 2 + (point.y - self.center_y) ** 2 <= self.radius ** 2
def geometry_type(self):
return "circle"
def intersects(self, other):
if isinstance(other, Circle):
return (self.center_x - other.center_x) ** 2 + (self.center_y - other.center_y) ** 2 <= (self.radius + other.radius) ** 2
elif isinstance(other, Line):
return other.intersects(self)
elif isinstance(other, Rectangle):
return other.intersects(self)
elif isinstance(other, Triangle):
return other.intersects(self)
elif isinstance(other, Polygon):
return other.intersects(self)
else:
raise ValueError("Invalid geometry type")
对于支持的简单几何文本格式,您可以编写一个解析器来解析这些文本,并使用 GeometryFactory 创建相应的几何形状。例如,对于 Circle 的文本格式,您可以编写如下的解析器:
def parse_circle(text):
parts = text.split()
center_x = float(parts[1])
center_y = float(parts[2])
radius = float(parts[3])
return factory.create_geometry("circle", center_x, center_y, radius)
其中,factory 是一个 GeometryFactory 的实例。对于其它几何形状的文本格式,您可以类似地编写解析器。
希望这个简单的几何工厂类的设计能够满足您的需求。
阅读全文
相关推荐















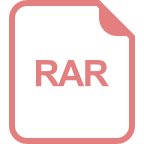
