逻辑回归代码实现带数据集的可视化代码
时间: 2023-07-26 11:40:50 浏览: 78
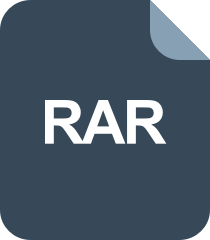
逻辑回归实现,包含数据集,源代码
好的,以下是一个简单的逻辑回归代码实现,并带有数据集的可视化。
```python
import numpy as np
import matplotlib.pyplot as plt
def sigmoid(z):
return 1 / (1 + np.exp(-z))
def cost_function(X, y, theta):
m = len(y)
h = sigmoid(X @ theta)
J = (-1/m) * (y.T @ np.log(h) + (1-y).T @ np.log(1-h))
grad = (1/m) * X.T @ (h-y)
return J, grad
def gradient_descent(X, y, theta, alpha, num_iters):
m = len(y)
J_history = []
for i in range(num_iters):
cost, grad = cost_function(X, y, theta)
theta = theta - (alpha * grad)
J_history.append(cost)
return theta, J_history
# 数据集
data = np.loadtxt('data.txt', delimiter=',')
X = data[:, :-1]
y = data[:, -1]
# 可视化数据
plt.scatter(X[:, 0], X[:, 1], c=y)
plt.xlabel('Exam 1 score')
plt.ylabel('Exam 2 score')
# 数据预处理
m, n = X.shape
X = np.concatenate([np.ones((m, 1)), X], axis=1)
initial_theta = np.zeros(n+1)
# 运行梯度下降算法
alpha = 0.01
num_iters = 10000
theta, J_history = gradient_descent(X, y, initial_theta, alpha, num_iters)
# 绘制决策边界
plot_x = np.array([np.min(X[:,1])-2, np.max(X[:,1])+2])
plot_y = (-1/theta[2]) * (theta[1]*plot_x + theta[0])
plt.plot(plot_x, plot_y)
plt.show()
```
其中,`data.txt` 是一个包含两个考试成绩和是否录取的数据集,可以自行准备。在运行代码后,会得到一个包含数据点和决策边界的可视化图形。
阅读全文
相关推荐
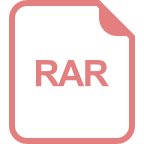
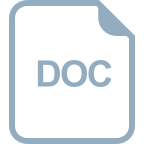
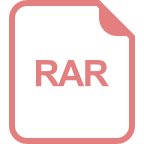
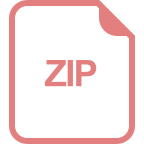
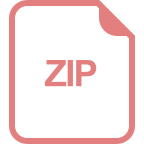
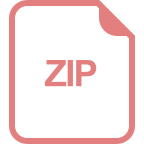
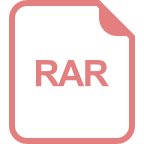
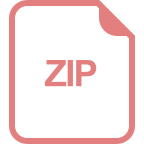
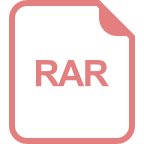
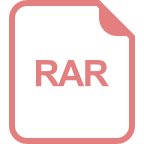
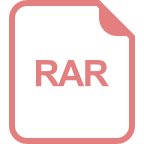
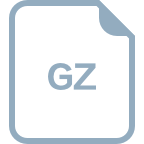
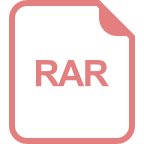
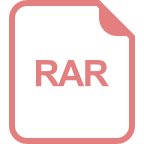
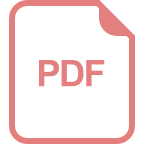
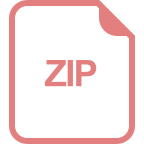