cshap 读取txt文件
时间: 2023-09-09 14:07:24 浏览: 107
以下是使用C#读取txt文件的示例代码:
```csharp
using System;
using System.IO;
class Program
{
static void Main(string[] args)
{
// 读取文件路径
string filePath = "text.txt";
try
{
// 打开文件流
using (StreamReader sr = new StreamReader(filePath))
{
// 逐行读取文件内容
string line;
while ((line = sr.ReadLine()) != null)
{
Console.WriteLine(line);
}
}
}
catch (Exception e)
{
Console.WriteLine(e.Message);
}
}
}
```
以上代码使用`StreamReader`类打开文件流,并逐行读取文件内容。如果发生异常,将会捕获并输出异常信息。
相关问题
cshap 读取TXT文件 判断包含ST字符后的第三行文字是否包含字母I
以下是C#代码示例,用于读取TXT文件并判断包含ST字符后的第三行文字是否包含字母I:
```csharp
using System;
using System.IO;
class Program
{
static void Main(string[] args)
{
string filePath = "example.txt";
bool containsI = false;
try
{
using (StreamReader sr = new StreamReader(filePath))
{
string line;
bool foundST = false;
int lineCount = 0;
while ((line = sr.ReadLine()) != null)
{
lineCount++;
if (line.Contains("ST"))
{
foundST = true;
}
else if (foundST && lineCount == 3)
{
if (line.Contains("I"))
{
containsI = true;
}
break;
}
}
}
if (containsI)
{
Console.WriteLine("The third line after 'ST' contains the letter 'I'.");
}
else
{
Console.WriteLine("The third line after 'ST' does not contain the letter 'I'.");
}
}
catch (Exception e)
{
Console.WriteLine("Error: " + e.Message);
}
Console.ReadLine();
}
}
```
在上面的代码中,我们首先定义了要读取的文件路径和一个布尔变量“containsI”,用于记录第三行是否包含字母“I”。然后,我们使用StreamReader打开文件,并使用ReadLine方法逐行读取文件内容。
在每一行中,我们检查该行是否包含“ST”字符,如果是,则将布尔变量“foundST”设置为true。一旦找到了“ST”,我们就开始计数,以便确定第三行。当计数到第三行时,我们检查该行是否包含字母“I”。如果是,则将布尔变量“containsI”设置为true,并立即退出while循环。
最后,我们检查“containsI”的值并输出相应的消息。请注意,我们还捕获了任何异常,并在控制台中输出错误消息。
cshap 读取编码格式为ANSI的txt文件
在C#中读取编码格式为ANSI的txt文件,可以使用StreamReader类,并设置编码为Encoding.Default。以下是示例代码:
```csharp
using System.IO;
using System.Text;
...
string filePath = "path/to/file.txt";
// 创建StreamReader对象,并设置编码为ANSI
StreamReader reader = new StreamReader(filePath, Encoding.Default);
// 逐行读取文件内容
string line;
while ((line = reader.ReadLine()) != null)
{
Console.WriteLine(line);
}
// 关闭流
reader.Close();
```
其中,Encoding.Default表示使用操作系统的默认编码,一般为ANSI编码。如果txt文件的编码不是ANSI,需要根据实际情况选择相应的编码。
阅读全文
相关推荐





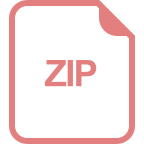
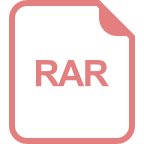




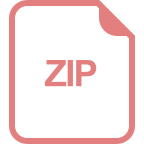