如何使用ssmo算法训练支持向量机,使用ssmo算法训练支持向量机的python代码是什么
时间: 2023-07-09 13:45:30 浏览: 54
SSMO算法是一种支持向量机的优化算法,可以用来寻找最优的超平面。以下是使用Python实现SSMO算法的代码示例:
```python
import numpy as np
class SVM:
def __init__(self, C=1.0, kernel='linear', tol=1e-3, max_iter=100):
self.C = C
self.kernel = kernel
self.tol = tol
self.max_iter = max_iter
def fit(self, X, y):
n_samples, n_features = X.shape
# Initialize alphas and biases
self.alpha = np.zeros(n_samples)
self.b = 0.0
# Calculate kernel matrix
self.K = np.zeros((n_samples, n_samples))
for i in range(n_samples):
for j in range(n_samples):
self.K[i,j] = self._kernel(X[i], X[j])
# Train SVM using SSMO algorithm
for _ in range(self.max_iter):
alpha_changed = 0
for i in range(n_samples):
E_i = self._predict(X[i]) - y[i]
if ((y[i]*E_i < -self.tol and self.alpha[i] < self.C) or
(y[i]*E_i > self.tol and self.alpha[i] > 0)):
j = self._select_second_alpha(i, n_samples)
E_j = self._predict(X[j]) - y[j]
alpha_i_old, alpha_j_old = self.alpha[i], self.alpha[j]
L, H = self._calculate_bounds(alpha_i_old, alpha_j_old, y[i], y[j])
if L == H:
continue
eta = self._calculate_eta(X[i], X[j])
if eta >= 0:
continue
self.alpha[j] = self._clip_alpha(alpha_j_old - (y[j]*(E_i - E_j))/eta, L, H)
if abs(self.alpha[j] - alpha_j_old) < self.tol:
continue
self.alpha[i] += y[i]*y[j]*(alpha_j_old - self.alpha[j])
b1 = self.b - E_i - y[i]*(self.alpha[i] - alpha_i_old)*self._kernel(X[i], X[i]) - y[j]*(self.alpha[j] - alpha_j_old)*self._kernel(X[i], X[j])
b2 = self.b - E_j - y[i]*(self.alpha[i] - alpha_i_old)*self._kernel(X[i], X[j]) - y[j]*(self.alpha[j] - alpha_j_old)*self._kernel(X[j], X[j])
if 0 < self.alpha[i] < self.C:
self.b = b1
elif 0 < self.alpha[j] < self.C:
self.b = b2
else:
self.b = (b1 + b2)/2
alpha_changed += 1
if alpha_changed == 0:
break
def _predict(self, X):
return np.sum(self.alpha * self.y * self.K[:,self.X] + self.b)
def _kernel(self, x1, x2):
if self.kernel == 'linear':
return np.dot(x1, x2)
elif self.kernel == 'rbf':
gamma = 0.1
return np.exp(-gamma*np.linalg.norm(x1-x2)**2)
def _select_second_alpha(self, i, n_samples):
j = i
while j == i:
j = np.random.randint(n_samples)
return j
def _calculate_bounds(self, alpha_i_old, alpha_j_old, y_i, y_j):
if y_i != y_j:
L = max(0, alpha_j_old - alpha_i_old)
H = min(self.C, self.C + alpha_j_old - alpha_i_old)
else:
L = max(0, alpha_i_old + alpha_j_old - self.C)
H = min(self.C, alpha_i_old + alpha_j_old)
return L, H
def _calculate_eta(self, x1, x2):
return 2*self._kernel(x1, x2) - self._kernel(x1, x1) - self._kernel(x2, x2)
def _clip_alpha(self, alpha, L, H):
if alpha < L:
return L
elif alpha > H:
return H
else:
return alpha
```
在使用时,可以通过以下代码进行训练:
```python
from sklearn.datasets import make_classification
from sklearn.model_selection import train_test_split
# Generate random dataset
X, y = make_classification(n_samples=100, n_features=10, n_informative=5, n_classes=2, random_state=1)
# Split dataset into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=1)
# Train SVM using SSMO algorithm
svm = SVM()
svm.fit(X_train, y_train)
# Test SVM on testing set
y_pred = np.sign([svm._predict(x) for x in X_test])
accuracy = np.mean(y_pred == y_test)
print('Accuracy:', accuracy)
```
相关推荐
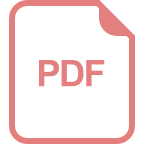
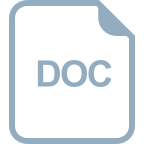
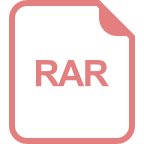
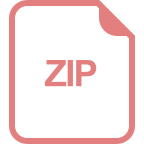
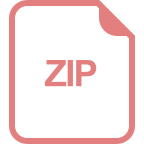
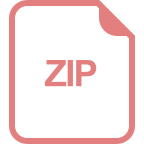
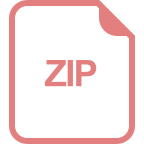
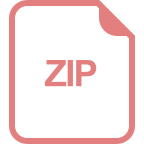
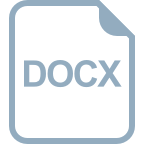
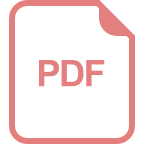