java里RSA加密怎么设置PKCS1
时间: 2023-05-23 15:05:56 浏览: 1047
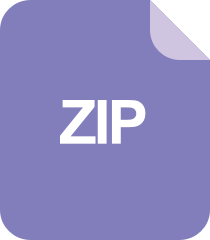
PKCS#1 java 语言实现参考 V1.0

Java中的RSA加密默认使用的是PKCS#1标准,可以通过以下代码设置为PKCS#1:
```
import java.security.*;
public class RSAEncryptor {
private static final String TRANSFORMATION = "RSA/ECB/PKCS1Padding";
public static void main(String[] args) throws Exception {
KeyPairGenerator keyGen = KeyPairGenerator.getInstance("RSA");
keyGen.initialize(2048);
KeyPair keyPair = keyGen.generateKeyPair();
PublicKey publicKey = keyPair.getPublic();
PrivateKey privateKey = keyPair.getPrivate();
Cipher cipher = Cipher.getInstance(TRANSFORMATION);
// 加密
cipher.init(Cipher.ENCRYPT_MODE, publicKey);
byte[] encryptedData = cipher.doFinal("Hello, world!".getBytes());
// 解密
cipher.init(Cipher.DECRYPT_MODE, privateKey);
byte[] decryptedData = cipher.doFinal(encryptedData);
System.out.println(new String(decryptedData));
}
}
```
其中,`TRANSFORMATION`常量设置为`RSA/ECB/PKCS1Padding`即可使用PKCS#1填充模式进行加密和解密。在`cipher.init()`方法中,第一个参数为加密模式或解密模式,第二个参数为密钥。在加密和解密时,需要分别使用公钥和私钥进行操作。
阅读全文
相关推荐

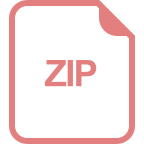
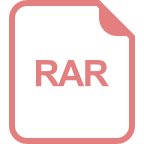
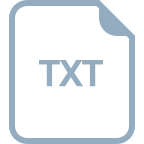
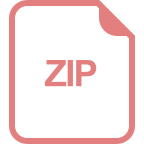
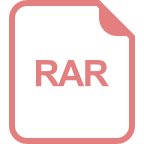
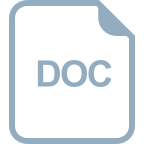




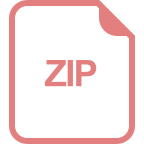
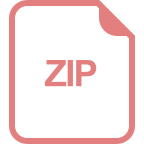
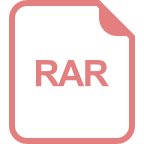
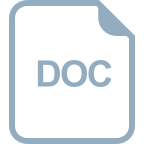
