java使用rsa加密解密
时间: 2023-07-07 09:28:14 浏览: 148
RSA算法是一种非对称加密算法,它可以用于加密数据和数字签名。在Java中,可以使用Java Cryptography Architecture(JCA)提供的API来实现RSA加密和解密。下面是简单的示例代码:
```java
import java.security.KeyPair;
import java.security.KeyPairGenerator;
import java.security.PrivateKey;
import java.security.PublicKey;
import java.security.SecureRandom;
import java.security.spec.PKCS8EncodedKeySpec;
import java.security.spec.X509EncodedKeySpec;
import javax.crypto.Cipher;
public class RSAEncryption {
public static void main(String[] args) throws Exception {
String plainText = "Hello World";
// 生成RSA公钥和私钥
KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance("RSA");
keyPairGenerator.initialize(2048, new SecureRandom());
KeyPair keyPair = keyPairGenerator.generateKeyPair();
PublicKey publicKey = keyPair.getPublic();
PrivateKey privateKey = keyPair.getPrivate();
// 使用公钥加密
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.ENCRYPT_MODE, publicKey);
byte[] cipherText = cipher.doFinal(plainText.getBytes());
// 使用私钥解密
cipher.init(Cipher.DECRYPT_MODE, privateKey);
byte[] decryptedText = cipher.doFinal(cipherText);
System.out.println("Plain Text: " + plainText);
System.out.println("Encrypted Text: " + new String(cipherText));
System.out.println("Decrypted Text: " + new String(decryptedText));
}
}
```
上面的代码先生成了一个2048位的RSA公钥和私钥,然后使用公钥加密了一个字符串,再使用私钥解密获得原始字符串。在实际使用中,我们通常需要将公钥和私钥保存在文件中,以便在不同的应用程序中使用。可以使用PKCS8EncodedKeySpec和X509EncodedKeySpec类来将公钥和私钥转换为字节数组,以便在文件中保存。
阅读全文
相关推荐
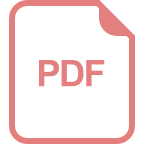
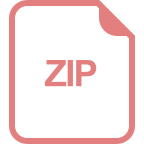
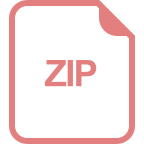















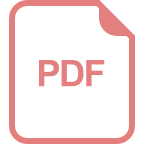