图片rsa加密解密java实现
时间: 2023-05-16 20:01:06 浏览: 260
RSA加密解密是公钥密码系统的重要方法之一,其通过生成公私钥对,实现加密数据的安全传输和解密数据的安全读取。图片RSA加密解密的实现同样依赖于该算法,其过程可分为以下几个步骤:
1. 图片转换:将待加密图片转换为二进制数据,
2. 公私钥生成:使用RSA算法生成公私钥对,其中公钥包含N和e两个参数,私钥包含N和d两个参数。
3. 加密:将二进制数据使用公钥进行加密,得到密文。
4. 解密:将密文使用私钥进行解密,得到二进制数据。
5. 数据还原:将解密后的二进制数据转换为图片。
Java语言提供了RSA加密解密的相关API,其中包含了上述步骤的实现方法。具体地,可以使用Java.security包下的KeyPairGenerator类生成公私钥对,使用Cipher类进行加密解密操作,使用Base64进行二进制数据的转换。
需要注意的是,图片RSA加密解密过程中可能会存在数据大小的限制,因此需要对较大的图片进行分块处理。同时,为了保证加密数据的安全,应该确保私钥的保密性。
相关问题
用Java语言设计并实现RSA加密解密程序,利用实现的RSA加密解密程序对文本加密和解密
以下是Java语言实现RSA加密解密程序的代码:
```java
import java.math.BigInteger;
import java.security.SecureRandom;
public class RSA {
private BigInteger n, d, e;
public RSA(int bitlen) {
SecureRandom r = new SecureRandom();
BigInteger p = new BigInteger(bitlen / 2, 100, r);
BigInteger q = new BigInteger(bitlen / 2, 100, r);
n = p.multiply(q);
BigInteger m = (p.subtract(BigInteger.ONE))
.multiply(q.subtract(BigInteger.ONE));
e = new BigInteger("3");
while (m.gcd(e).intValue() > 1) {
e = e.add(new BigInteger("2"));
}
d = e.modInverse(m);
}
public BigInteger encrypt(BigInteger message) {
return message.modPow(e, n);
}
public BigInteger decrypt(BigInteger message) {
return message.modPow(d, n);
}
public static void main(String[] args) {
RSA rsa = new RSA(1024);
BigInteger message = new BigInteger("123456789");
BigInteger encrypted = rsa.encrypt(message);
BigInteger decrypted = rsa.decrypt(encrypted);
System.out.println("Original message: " + message);
System.out.println("Encrypted message: " + encrypted);
System.out.println("Decrypted message: " + decrypted);
}
}
```
该程序使用了Java的BigInteger类来处理大数运算。在构造函数中,随机生成两个大素数p和q,并计算n和m。然后选择一个小于m的整数e作为加密密钥,计算d作为解密密钥。encrypt和decrypt方法分别实现了RSA的加密和解密算法。在main方法中,使用生成的密钥对一个数字进行加密和解密,并输出结果。
要使用该程序对文本进行加密和解密,可以将文本转换为BigInteger类型,然后调用encrypt和decrypt方法。以下是一个简单的示例:
```java
import java.math.BigInteger;
public class RSAExample {
public static void main(String[] args) {
RSA rsa = new RSA(1024);
String message = "Hello, world!";
BigInteger plaintext = new BigInteger(message.getBytes());
BigInteger encrypted = rsa.encrypt(plaintext);
BigInteger decrypted = rsa.decrypt(encrypted);
System.out.println("Original message: " + message);
System.out.println("Encrypted message: " + encrypted);
System.out.println("Decrypted message: " + new String(decrypted.toByteArray()));
}
}
```
该程序将字符串"Hello, world!"转换为字节数组,然后将其转换为BigInteger类型并加密。解密后再将结果转换为字节数组,最终转换为字符串输出。
java实现rsa加密解密
Java可以通过使用Java Cryptography Architecture(JCA)提供的API来实现RSA加密和解密。下面是一个简单的实现步骤:
1. 生成公钥和私钥对,可以使用KeyPairGenerator类来生成。
2. 使用公钥加密明文,可以使用Cipher类来实现。
3. 使用私钥解密密文,同样可以使用Cipher类来实现。
下面是一个简单的Java代码示例:
```
import java.security.*;
import javax.crypto.*;
public class RSAExample {
public static void main(String[] args) throws Exception {
// 生成公钥和私钥对
KeyPairGenerator keyGen = KeyPairGenerator.getInstance("RSA");
keyGen.initialize(2048);
KeyPair keyPair = keyGen.generateKeyPair();
PublicKey publicKey = keyPair.getPublic();
PrivateKey privateKey = keyPair.getPrivate();
// 使用公钥加密明文
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.ENCRYPT_MODE, publicKey);
byte[] plaintext = "Hello, world!".getBytes();
byte[] ciphertext = cipher.doFinal(plaintext);
// 使用私钥解密密文
cipher.init(Cipher.DECRYPT_MODE, privateKey);
byte[] decrypted = cipher.doFinal(ciphertext);
String decryptedText = new String(decrypted);
System.out.println(decryptedText);
}
}
```
该代码生成了一个2048位的RSA密钥对,并使用公钥加密了一个字符串,然后使用私钥解密了密文并打印出明文。
阅读全文
相关推荐
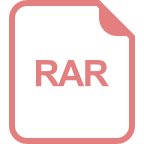
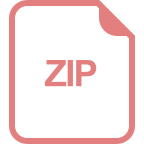
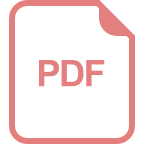
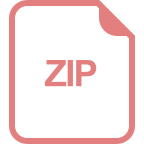
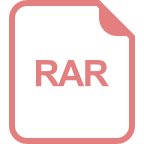
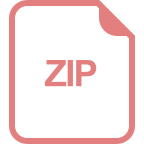
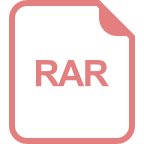
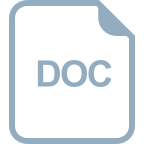
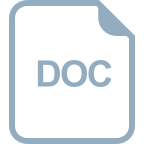