/** * 论坛详情 */ @IgnoreAuth @RequestMapping("/list/{id}") public R list(@PathVariable("id") String id){ ForumEntity forum = forumService.selectById(id); getChilds(forum); return R.ok().put("data", forum); } private ForumEntity getChilds(ForumEntity forum) { List<ForumEntity> childs = new ArrayList<ForumEntity>(); childs = forumService.selectList(new EntityWrapper<ForumEntity>().eq("parentid", forum.getId())); if(childs == null || childs.size()==0) { return null; } forum.setChilds(childs); for(ForumEntity forumEntity : childs) { getChilds(forumEntity); } return forum; }
时间: 2024-01-02 21:01:48 浏览: 55
这段代码是一个Java后端接口实现,用于获取论坛的详情信息。@IgnoreAuth注解表示该接口不需要进行身份验证即可访问。@PathVariable注解用于获取请求路径中的id参数,并将其作为参数传递给list()方法。list()方法首先通过id查询获取到对应的ForumEntity对象,然后调用getChilds()方法获取该论坛的子论坛列表,并将其设置到ForumEntity对象的childs属性中。getChilds()方法通过递归调用获取所有子论坛的列表,并将它们设置到父论坛的childs属性中。最后,该接口返回一个响应对象R,其中包含查询到的论坛详情信息。
相关问题
解释这段代码@RequestMapping("config") @RestController public class ConfigController{ @Autowired private ConfigService configService; /** * 列表 */ @RequestMapping("/page") public R page(@RequestParam Map<String, Object> params,ConfigEntity config){ EntityWrapper<ConfigEntity> ew = new EntityWrapper<ConfigEntity>(); PageUtils page = configService.queryPage(params); return R.ok().put("data", page); } /** * 列表 */ @IgnoreAuth @RequestMapping("/list") public R list(@RequestParam Map<String, Object> params,ConfigEntity config){ EntityWrapper<ConfigEntity> ew = new EntityWrapper<ConfigEntity>(); PageUtils page = configService.queryPage(params); return R.ok().put("data", page); } /** * 信息 */ @RequestMapping("/info/{id}") public R info(@PathVariable("id") String id){ ConfigEntity config = configService.selectById(id); return R.ok().put("data", config); } /** * 详情 */ @IgnoreAuth @RequestMapping("/detail/{id}") public R detail(@PathVariable("id") String id){ ConfigEntity config = configService.selectById(id); return R.ok().put("data", config); } /** * 根据name获取信息 */ @RequestMapping("/info") public R infoByName(@RequestParam String name){ ConfigEntity config = configService.selectOne(new EntityWrapper<ConfigEntity>().eq("name", "faceFile")); return R.ok().put("data", config); } /** * 保存 */ @PostMapping("/save") public R save(@RequestBody ConfigEntity config){ // ValidatorUtils.validateEntity(config); configService.insert(config); return R.ok(); } /** * 修改 */ @RequestMapping("/update") public R update(@RequestBody ConfigEntity config){ // ValidatorUtils.validateEntity(config); configService.updateById(config);//全部更新 return R.ok(); } /** * 删除 */ @RequestMapping("/delete") public R delete(@RequestBody Long[] ids){ configService.deleteBatchIds(Arrays.asList(ids)); return R.ok(); } }
这段代码是一个 Spring Boot 的 RESTful API 接口,使用了 Spring MVC 的注解来定义 URL 路由和 HTTP 请求方法。该接口包含了对 "config" 实体的增删改查操作。具体说明如下:
1. `@RequestMapping("config")`:定义了该接口的基本路径为 "/config"。
2. `@RestController`:声明该类为控制器,同时该类中的所有方法都会返回 JSON 格式的数据。
3. `@Autowired`:自动注入 ConfigService 对象。
4. `@RequestMapping("/page")` 和 `@RequestMapping("/list")`:定义了两个接口,分别用于分页查询和列表查询。
5. `@IgnoreAuth`:忽略授权,即不需要登录即可访问。
6. `@RequestMapping("/info/{id}")` 和 `@RequestMapping("/detail/{id}")`:定义了两个接口,用于获取单条记录的详细信息。
7. `@RequestMapping("/info")`:定义了一个接口,用于根据名称获取单条记录的详细信息。
8. `@PostMapping("/save")`:定义了一个接口,用于新增一条记录。
9. `@RequestMapping("/update")`:定义了一个接口,用于修改一条记录。
10. `@RequestMapping("/delete")`:定义了一个接口,用于删除多条记录。
/** * 前端列表 */ @IgnoreAuth @RequestMapping("/list") public R list(@RequestParam Map<String, Object> params,ZhuxuejinEntity zhuxuejin, HttpServletRequest request){ EntityWrapper<ZhuxuejinEntity> ew = new EntityWrapper<ZhuxuejinEntity>(); PageUtils page = zhuxuejinService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, zhuxuejin), params), params)); return R.ok().put("data", page); } /** * 列表 */ @RequestMapping("/lists") public R list( ZhuxuejinEntity zhuxuejin){ EntityWrapper<ZhuxuejinEntity> ew = new EntityWrapper<ZhuxuejinEntity>(); ew.allEq(MPUtil.allEQMapPre( zhuxuejin, "zhuxuejin")); return R.ok().put("data", zhuxuejinService.selectListView(ew)); }
这段代码是一个Java后台的控制器类,其中包含了两个处理请求的方法。第一个方法的请求路径是“/list”,它使用了一个名为“params”的Map类型参数和一个名为“zhuxuejin”的ZhuxuejinEntity类型参数来获取请求参数。方法中使用了EntityWrapper类对zhuxuejin进行了条件查询,并使用PageUtils类进行分页处理,最后将查询结果返回。
第二个方法的请求路径是“/lists”,它只接受一个名为“zhuxuejin”的ZhuxuejinEntity类型参数,并使用EntityWrapper类对zhuxuejin进行了条件查询,最后将查询结果返回。
另外,这两个方法中使用了@IgnoreAuth和@RequestMapping注解进行请求映射和权限控制。
相关推荐
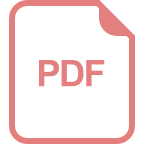
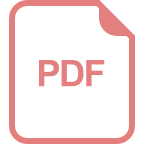
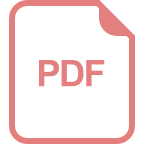










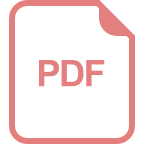
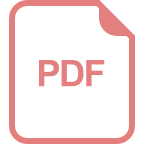
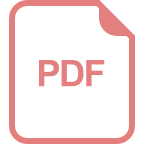