请帮我写一个贪吃蛇游戏的代码
时间: 2023-06-04 11:04:56 浏览: 88
好的,以下是一个简单的贪吃蛇游戏的代码示例,使用Python语言编写:
```python
import pygame
import random
pygame.init()
window_size = (500, 500)
cell_size = 20
grid_size = (window_size[0] // cell_size, window_size[1] // cell_size)
window = pygame.display.set_mode(window_size)
pygame.display.set_caption("贪吃蛇游戏")
clock = pygame.time.Clock()
font = pygame.font.Font(None, 30)
def draw_grid():
for x in range(0, window_size[0], cell_size):
pygame.draw.line(window, (128, 128, 128), (x, 0), (x, window_size[1]))
for y in range(0, window_size[1], cell_size):
pygame.draw.line(window, (128, 128, 128), (0, y), (window_size[0], y))
class Snake:
def __init__(self):
self.body = [(0, 0), (1, 0), (2, 0)]
self.direction = "RIGHT"
self.score = 0
self.is_alive = True
def move(self):
head_x, head_y = self.body[0]
if self.direction == "UP":
new_head = (head_x, head_y-1)
elif self.direction == "DOWN":
new_head = (head_x, head_y+1)
elif self.direction == "LEFT":
new_head = (head_x-1, head_y)
elif self.direction == "RIGHT":
new_head = (head_x+1, head_y)
if new_head in self.body[1:]:
self.is_alive = False
if new_head[0] < 0 or new_head[0] >= grid_size[0] or new_head[1] < 0 or new_head[1] >= grid_size[1]:
self.is_alive = False
self.body.insert(0, new_head)
if new_head == food.position:
self.score += 1
food.spawn()
else:
self.body.pop()
def draw(self):
for x, y in self.body:
rect = pygame.Rect(x*cell_size, y*cell_size, cell_size, cell_size)
pygame.draw.rect(window, (0, 255, 0), rect)
text = font.render("得分: {}".format(self.score), True, (255, 255, 255))
window.blit(text, (10, 10))
class Food:
def __init__(self):
self.spawn()
def spawn(self):
self.position = (random.randint(0, grid_size[0]-1), random.randint(0, grid_size[1]-1))
def draw(self):
rect = pygame.Rect(self.position[0]*cell_size, self.position[1]*cell_size, cell_size, cell_size)
pygame.draw.rect(window, (255, 0, 0), rect)
snake = Snake()
food = Food()
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP and snake.direction != "DOWN":
snake.direction = "UP"
elif event.key == pygame.K_DOWN and snake.direction != "UP":
snake.direction = "DOWN"
elif event.key == pygame.K_LEFT and snake.direction != "RIGHT":
snake.direction = "LEFT"
elif event.key == pygame.K_RIGHT and snake.direction != "LEFT":
snake.direction = "RIGHT"
if not snake.is_alive:
text = font.render("游戏结束,得分: {}".format(snake.score), True, (255, 255, 255))
window.blit(text, (window_size[0]//2-text.get_width()//2, window_size[1]//2-text.get_height()//2))
pygame.display.update()
pygame.time.delay(1500)
break
window.fill((0, 0, 0))
draw_grid()
food.draw()
snake.move()
snake.draw()
pygame.display.update()
clock.tick(10)
pygame.quit()
```
这个代码示例只是一个非常简单的贪吃蛇游戏,有很多可以改进的地方,如增加难度、添加音效等等。如果你想深入了解如何开发游戏或使用Python进行编程,请通过其他渠道获取更多信息和教程。
阅读全文
相关推荐





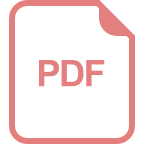
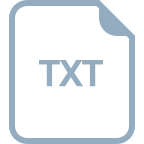
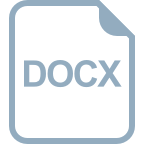
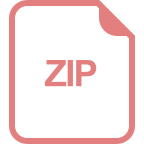
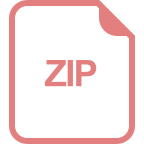
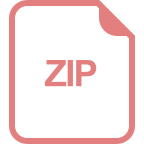