HBase查询行键大于等于543210的所有数据
时间: 2024-03-08 22:49:51 浏览: 27
您可以使用HBase的Scan API来查询行键大于等于543210的所有数据。具体实现可以参考以下Java代码:
```java
Configuration config = HBaseConfiguration.create();
Connection connection = ConnectionFactory.createConnection(config);
Table table = connection.getTable(TableName.valueOf("your_table_name"));
Scan scan = new Scan();
scan.setStartRow(Bytes.toBytes("543210"));
ResultScanner scanner = table.getScanner(scan);
for (Result result : scanner) {
// 处理查询结果
}
scanner.close();
table.close();
connection.close();
```
该代码会创建一个HBase连接,并获取名为“your_table_name”的表。然后,它使用Scan对象设置起始行键为543210,通过table.getScanner(scan)方法获取符合条件的所有数据。最后,您可以通过for循环遍历查询结果,进行进一步的处理。
相关问题
hbase查询行键为jack_course的数据
你可以使用HBase的命令行界面或者是编写Java代码来查询行键为jack_course的数据。以下是使用命令行界面进行查询的步骤:
1. 打开HBase的shell:`hbase shell`
2. 进入需要查询的表:`scan 'table_name'`
3. 查询行键为jack_course的数据:`get 'table_name', 'jack_course'`
如果你使用Java代码进行查询,可以参考以下示例代码:
```
Configuration config = HBaseConfiguration.create();
Connection connection = ConnectionFactory.createConnection(config);
Table table = connection.getTable(TableName.valueOf("table_name"));
Get get = new Get(Bytes.toBytes("jack_course"));
Result result = table.get(get);
```
这段代码会查询名为"table_name"的表中行键为"jack_course"的数据,并将结果保存在Result对象中。
hbase的行键型数据是什么样的数据
HBase是一个列式数据库,它的数据存储是基于行键(Row Key)的。行键型数据是指按照行键来存储和组织数据的数据类型。在HBase中,每个数据行都有一个唯一的行键,行键可以是任何可序列化的字节数组。HBase将数据按照行键进行排序并存储在HDFS上,因此可以通过行键来快速查找和访问数据。HBase中的数据可以根据行键的前缀范围进行扫描,这使得HBase非常适合存储大量按照时间序列排列的数据,例如日志数据、传感器数据、金融交易数据等。
相关推荐
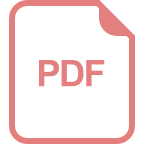
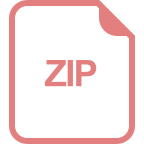












