C++根据经度,纬度,速度(节)和航向计算坐标的代码实现
时间: 2023-09-20 07:09:10 浏览: 196
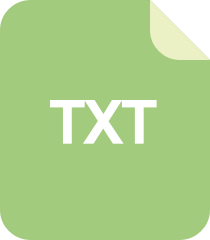
一个经纬度相关计算的C++类
以下是一个简单的C++函数,可以根据经度、纬度、速度和航向计算出当前位置的坐标:
```c++
#include <cmath>
const double pi = acos(-1.0);
const double R = 6371.0; // 地球半径,单位为千米
// 计算角度的弧度值
double rad(double d)
{
return d * pi / 180.0;
}
// 计算两点之间的距离,单位为千米
double get_distance(double lat1, double lng1, double lat2, double lng2)
{
double rad_lat1 = rad(lat1);
double rad_lat2 = rad(lat2);
double a = rad_lat1 - rad_lat2;
double b = rad(lng1) - rad(lng2);
double s = 2 * asin(sqrt(pow(sin(a / 2), 2) + cos(rad_lat1) * cos(rad_lat2) * pow(sin(b / 2), 2)));
s *= R;
return s;
}
// 根据经度、纬度、速度和航向计算下一个位置的坐标
void get_next_position(double lat, double lng, double speed, double heading, double& next_lat, double& next_lng)
{
double distance = speed / 3600.0; // 将速度从节转换为千米每秒
double rad_heading = rad(heading);
double dx = distance * sin(rad_heading);
double dy = distance * cos(rad_heading);
double lat_radius = R * cos(rad(lat)); // 纬度圆的半径
next_lat = lat + (dy / R) * 180.0 / pi; // 计算下一个位置的纬度
next_lng = lng + (dx / lat_radius) * 180.0 / pi; // 计算下一个位置的经度
}
```
使用方法:
```c++
double lat = 30.0; // 当前位置的纬度
double lng = 120.0; // 当前位置的经度
double speed = 10.0; // 当前速度,单位为节
double heading = 45.0; // 当前航向,单位为度
double next_lat, next_lng;
get_next_position(lat, lng, speed, heading, next_lat, next_lng); // 计算下一个位置的坐标
```
这个函数中,`get_distance`函数用于计算两点之间的距离,`get_next_position`函数用于根据当前位置、速度和航向计算下一个位置的坐标。其中,`next_lat`和`next_lng`是输出参数,用于保存计算出的下一个位置的纬度和经度。
阅读全文
相关推荐
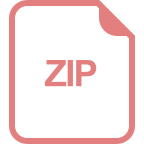
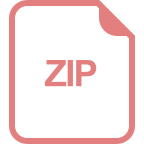
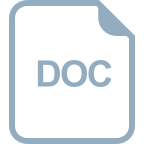
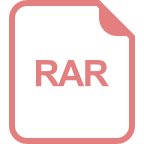
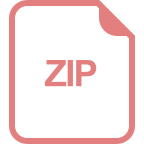
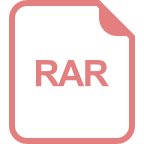
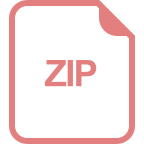
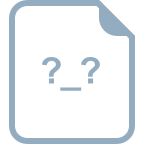
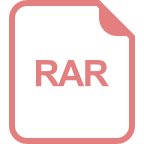
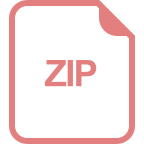
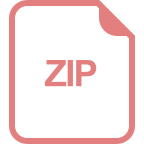
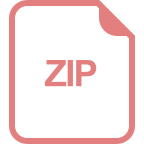
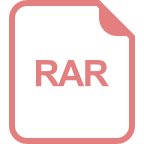

